The easiest approach is to bind the column count to the text length, but it won’t look very accurate, since a TextField uses its column count to account for the widest characters of the font:
iterations.prefColumnCountProperty().bind(
Bindings.max(2, iterations.lengthProperty()));
(Looks pretty good if you only type W
characters.)
A better-looking, but more complicated, solution is to use the TextField’s skin to figure out the proper size:
import javafx.application.Application;
import javafx.application.Platform;
import javafx.geometry.Insets;
import javafx.stage.Stage;
import javafx.scene.Scene;
import javafx.scene.control.TextField;
import javafx.scene.control.skin.TextFieldSkin;
import javafx.scene.layout.HBox;
public class GrowingTextField
extends Application {
private double margin = -1;
private double minWidth = -1;
@Override
public void start(Stage stage) {
TextField iterations = new TextField(" ");
iterations.setPrefColumnCount(2);
iterations.skinProperty().addListener((o, old, skin) -> {
if (skin != null && margin < 0) {
TextFieldSkin textFieldSkin = (TextFieldSkin) skin;
Platform.runLater(() -> {
margin = textFieldSkin.getCharacterBounds(0).getMinX();
minWidth = iterations.prefWidth(0);
Platform.runLater(() -> iterations.setText(""));
});
}
});
iterations.textProperty().addListener((o, oldText, text) -> {
double width;
if (text.length() <= iterations.getPrefColumnCount()) {
width = minWidth;
} else {
TextFieldSkin skin = (TextFieldSkin) iterations.getSkin();
double left = skin.getCharacterBounds(0).getMinX();
double right = skin.getCharacterBounds(
text.offsetByCodePoints(text.length(), -1)).getMaxX();
width = right - left + margin * 2;
}
Platform.runLater(() -> iterations.setPrefWidth(width));
});
HBox pane = new HBox(iterations);
pane.setPadding(new Insets(12));
stage.setScene(new Scene(pane, 800, 150));
stage.setTitle("Growing TextField");
stage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Output
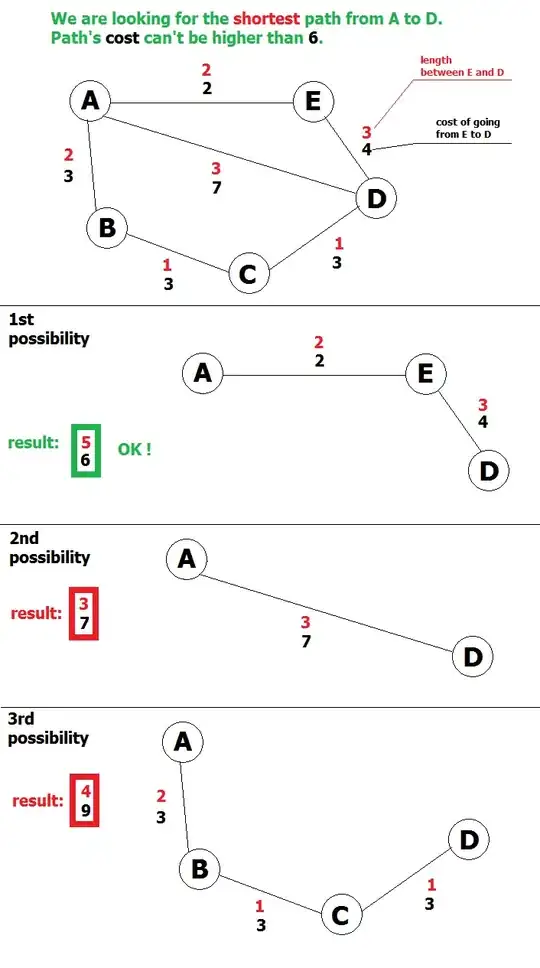
I do question why one would need this. Why not just give the TextField a large column count to start with, like a web browser’s URL field?