I used below c# code in my Azure HTTP Trigger Function to get Azure Service principal properties with client credentials flow and it was successful, Refer below:-
My HTTP Trigger Function.cs code:-
I modified the below code from my SO thread answer here to use graph API in Functions.
using Azure.Core;
using Azure.Identity;
using Newtonsoft.Json;
using System;
using System.Net.Http;
using System.Threading.Tasks;
using Azure.Core;
using Azure.Identity;
using Newtonsoft.Json;
using System;
using System.Net.Http;
using System.Threading.Tasks;
using Microsoft.AspNetCore.Http;
using Microsoft.AspNetCore.Mvc;
using Microsoft.Azure.WebJobs;
using Microsoft.Azure.WebJobs.Extensions.Http;
using Microsoft.Extensions.Logging;
using System.Net.Http.Json;
namespace FunctionApp1
{
public static class Function1
{
[FunctionName("Function1")]
public static async Task<IActionResult> Run(
[HttpTrigger(AuthorizationLevel.Function, "get", Route = null)] HttpRequest req,
ILogger log)
{
log.LogInformation("C# HTTP trigger function processed a request.");
var token = await GetAccessToken("<tenant-id>", "<client-id>", "<client-secret>");
var results = await GetResults(token);
return new OkObjectResult(results);
}
private static async Task<string> GetAccessToken(string tenantId, string clientId, string clientKey)
{
var credentials = new ClientSecretCredential(tenantId, clientId, clientKey);
var result = await credentials.GetTokenAsync(new TokenRequestContext(new[] { "https://graph.microsoft.com/.default"
}), default);
return result.Token;
}
private static async Task<string> GetResults(string token)
{
var httpClient = new HttpClient
{
BaseAddress = new Uri("https://graph.microsoft.com/v1.0/")
};
string URI = $"applications/7297aaca-505f-48c2-9670-3cad6fad99e7";
httpClient.DefaultRequestHeaders.Remove("Authorization");
httpClient.DefaultRequestHeaders.Add("Authorization", "Bearer " + token);
HttpResponseMessage response = await httpClient.GetAsync(URI);
var HttpsResponse = await response.Content.ReadAsStringAsync();
//var JSONObject = JsonConvert.DeserializeObject<object>(HttpsResponse);
//return response.StatusCode.ToString();
return HttpsResponse;
}
}
}
Local Output:-
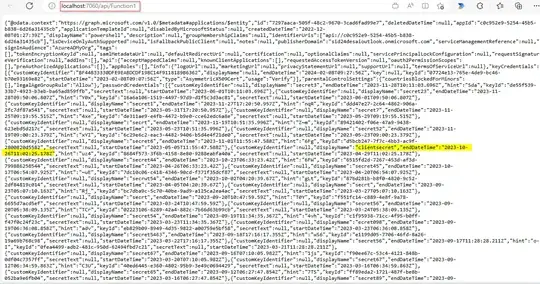
I deployed the Function in Azure Function app and got the desired results, Refer below:-
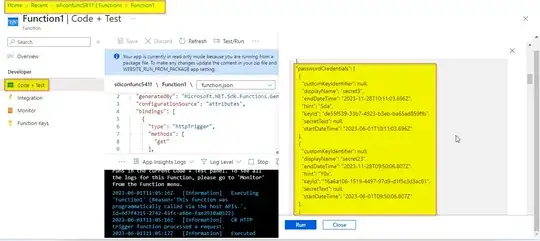
Reference:-
https://learn.microsoft.com/en-us/graph/api/serviceprincipal-get?view=graph-rest-1.0&tabs=http#code-try-1