This would be a good use-case for look aheads, since that's how you're naturally defining when the string should end. Since you want either the end of the string ($) or a doublquote (") - but whichever comes first, you could get away with using something like:
msg="(.*?)(?="|$)
Where the capture group is the contents of the msg field. Chris Maurer's answer is very important -- the .*? vs .* makes the behavior lazy//stop as soon as possible. Otherwise, it would default to continuing to end of line. However, if there are many lines you are looking through, you would either need to make this a multi-line flagged regex (which is slow), or add in a check for end of line rather than end of string. That would look like this:
msg="(.*?)(?="|\n|$)
Checked in Regex101 and it looks to match both cases. Best of luck! To reiderate, though, Chris hit the nail on the head- you not only need to be careful with your greedy/lazy quantifer but also escaping that " out. Most langauages use \" to do that.
EDIT: @chepner brought up a good point. If there is a " in the msg field, it'll throw your regex way off. Instead, we can check for either end of line/string OR just the next tag! Here's what I came up with for that:
msg=\"(.*?)(?=\" [a-z]+=|\n|$)
Assuming all the tags are lowercase a-z's and followed by =" then this should work just fine, as seen here:
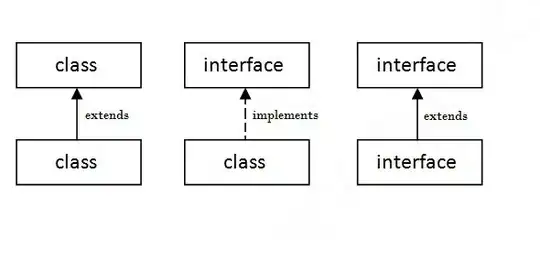