This can be achieved by editing the plot and axis margin, and arranging the plots using ggpubr
:
library(ggplot2)
library(ggpubr)
test_data <-
data.frame(
sample = c("label a", "label b", "label c", "label d", "label e", "label f"),
var1 = c(20, 24, 19, 21, 45, 23),
var2 = c(4000, 3890, 4020, 3760, 4322, 5434)
)
axis_margin <- 5.5
p1 <- ggplot(test_data, aes(var1, sample)) +
geom_col() +
scale_x_reverse() +
scale_y_discrete(position = "right") +
theme(
axis.text.y = element_blank(),
axis.title.y = element_blank(),
plot.margin = margin(axis_margin, 0, axis_margin, axis_margin)
)
p2 <- ggplot(test_data, aes(var2, sample)) +
geom_col() +
theme(
axis.title.y = element_blank(),
plot.margin = margin(axis_margin, axis_margin, axis_margin, 0),
axis.text.y.left = element_text(margin = margin(0, axis_margin, 0, axis_margin))
)
ggarrange(p1, p2)
For the left plot, you need to hide the y-axis labels and title. Set the axis position to "right" for the ticks to be in the correct position. Then you need to remove the right margin for the plot (default is 5.5). Also, you need to flip the x-axis.
For the right plot, you remove the y-axis title and remove the left margin of the plot. The distance between the two plots is controlled by setting the margin of the axis.text.y.left
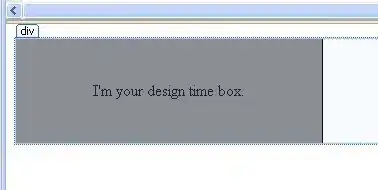