Try this code!!!
Solution 1:
Output
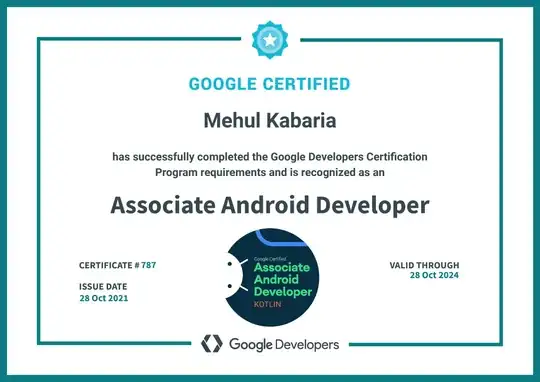
import 'package:flutter/material.dart';
class InvertedTriangle extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
body: Column(
crossAxisAlignment: CrossAxisAlignment.center,
mainAxisAlignment: MainAxisAlignment.center,
children: [
Container(
height: 50,
width: 350,
alignment: Alignment.bottomCenter,
decoration: const BoxDecoration(
borderRadius: BorderRadius.only(
topRight: Radius.circular(12),
topLeft: Radius.circular(12),
),
color: Colors.blue,
),
child: const Text(
"Lorem Ipsum",
style: TextStyle(
color: Colors.white,
),
),
),
CustomPaint(
painter: InvertedTrianglePainter(),
size: Size(350, 350),
),
],
),
);
}
}
class InvertedTrianglePainter extends CustomPainter {
@override
void paint(Canvas canvas, Size size) {
final double width = size.width;
final double height = size.height;
final double bluntHeight =
height * 0.19; // Adjust the blunt height as per your requirement
final double bluntOffset =
width * 0.022; // Adjust the blunt offset as per your requirement
final path = Path();
path.moveTo(0, 0); // Top left corner
path.lineTo(width, 0); // Top right corner
path.lineTo(width / 2 + bluntOffset, bluntHeight); // Top blunt corner
path.quadraticBezierTo(
width / 2,
bluntHeight * 1.06,
width / 2 - bluntOffset,
bluntHeight,
); // Curve the tip of the triangle
path.lineTo(0, 0); // Top left corner
path.close();
final paint = Paint()..color = Colors.blue;
canvas.drawPath(path, paint);
}
@override
bool shouldRepaint(CustomPainter oldDelegate) {
return false;
}
}
void main() {
runApp(MaterialApp(
home: InvertedTriangle(),
));
}
Solution 2:
import 'package:flutter/material.dart';
class InvertedTriangle extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Inverted Triangle'),
),
body: Center(
child: CustomPaint(
painter: InvertedTrianglePainter(),
size: Size(500, 500), // Adjust the size as per your requirement
),
),
);
}
}
class InvertedTrianglePainter extends CustomPainter {
@override
void paint(Canvas canvas, Size size) {
final double width = size.width;
final double height = size.height;
final double bluntHeight = height * 0.2; // Adjust the blunt height as per your requirement
final double bluntOffset = width * 0.01; // Adjust the blunt offset as per your requirement
final path = Path();
path.moveTo(0, 0); // Top left corner
path.lineTo(width, 0); // Top right corner
path.lineTo(width / 2 + bluntOffset, bluntHeight); // Top blunt corner
path.lineTo(width / 2 - bluntOffset, bluntHeight); // Top blunt corner
path.lineTo(0, 0); // Top left corner
path.close();
final paint = Paint()..color = Colors.blue;
canvas.drawPath(path, paint);
}
@override
bool shouldRepaint(CustomPainter oldDelegate) {
return false;
}
}
void main() {
runApp(MaterialApp(
home: InvertedTriangle(),
));
}