This can be done using pandas only.
The data tables have the following attributes
{'border':'1', 'width':'100%', 'align':'center', 'cellspacing':'0', 'cellpadding':'2', 'bgcolor':'#E8E8E8', 'bordercolor':'#E8E8E8'}
Using these, we can filter out all the tables from the webpage, clean the data and save it to an excel file.
import pandas as pd
categories = ['MANAGEMENT','ENGINEERING','MEDICAL','LAW','UPSC','SSC','SCIENCE','DESIGN','UGC','BANK TEST','OTHER ENTRANCE EXAMS']
# fetch all tables from the webpage with given attributes
tables = pd.read_html('https://career.webindia123.com/career/exams/examdate.asp', attrs={'border':'1', 'width':'100%', 'align':'center', 'cellspacing':'0', 'cellpadding':'2', 'bgcolor':'#E8E8E8', 'bordercolor':'#E8E8E8'})
columns = ['Exam Date', 'Last Date of Application', 'Examination']
for _ in range(len(tables)):
tables[_].columns = columns # assign columns to each table
tables[_] = tables[_][tables[_]['Exam Date'] != 'Completed Applications'] #filter rows which have *Completed Applications* in text, as those are blank rows
tables[_].insert(len(tables[_].columns), 'Category', categories[_]) #add category to each row
tables = pd.concat(tables, ignore_index=True)
tables.to_csv('Data.csv', index=None)
With this code, I am filtering unneeded rows and assigning the category (of the exam) to the data as well then saving it to a CSV file.
Following is a sample of data from the CSV file.
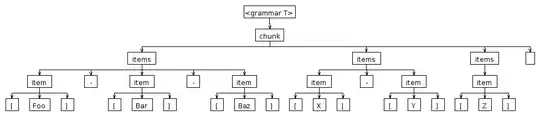