The FirstOrDefaultAsync
method is used to retrieve the first element that satisfies the specified criteria, or the default value if no such element is found. It is a query method that does not involve modifying the state of the entity or instructing EF to track the entity.
AddAsync()
is 100% async safe, while Add()
is only async safe in certain conditions.
For details, you can refer to AddAsync() vs Add() in EF Core
None of these are directly related to tracking a given entity.
For tracking a given entity, you can pay more attention to SaveChanges
, the following is an example, by catching DbUpdateException
to track entities and fields, because DbUpdateException
is usually an error reported in SaveChanges
:
try
{
using (var context = new EfContext())
{
var data = new TestTable
{
Status = TestTableStatus.Status1.ToString(),
Content = "One-to-many test",
TestTableItems = new List<TestTableItem> {
new TestTableItem { Name = "Add one-to-many 1", Id = 123},
new TestTableItem {Name = "Add one-to-many 2" } }
};
context. Add(data);
await context. SaveChangesAsync();
}
}
catch (DbUpdateException ex)
{
var failedEntries = ex.Entries;
foreach (var entry in failedEntries)
{
var entityName = entry. Metadata. Name;
Console.WriteLine($"Failed to add in entity: {entityName}");
}
}
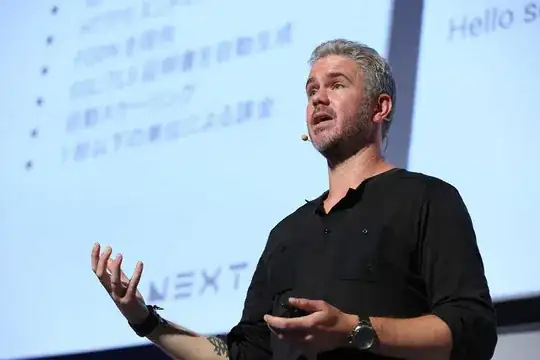