It looks like the server that is running locally is already in use.
You can refer this SO thread answer by Bartosz
Add this line of code to resuse the same address in your code that is running the server:-
udp = socket.socket(socket.AF_INET,
socket.SOCK_DGRAM)
udp.bind((UDP_IP, UDP_PORT))
udp.setsockopt(socket.SOL_SOCKET, socket.SO_REUSEADDR, 1)
Another option is to kill the process of running address according to the answer by MohitGhodasara, Ayub laziz and Abdul Basit:-
$ ps -fA | grep python 501 81211 12368 0 10:11PM ttys000 0:03.12
python -m SimpleHTTPServer
$ kill 81211
My apply_model_server.py code:-
from flask import Flask, request
app = Flask(__name__)
@app.route('/')
def root():
return "Welcome to the Voice Cloning API"
@app.route('/apply_model', methods=['POST'])
def apply_model():
# Get the audio data and model name from the request
audio_data = request.json['audio']
model_name = request.json['model_name']
# Apply the specified model on the audio data
processed_audio = process_audio(audio_data, model_name)
# Return the processed audio as the response
return processed_audio
def process_audio(audio_data, model_name):
# Your code to apply the model on the audio goes here
# Implement the logic to apply the specified model on the audio data
# Example: You can use your voice cloning model here
# Replace the following line with your actual model application logic
processed_audio = f"Model: {model_name}, Audio: {audio_data}"
return processed_audio
if __name__ == '__main__':
app.run(debug=True)
My apply_model server is running on a different port in local host and my function trigger is running on a different host, Refer below:-
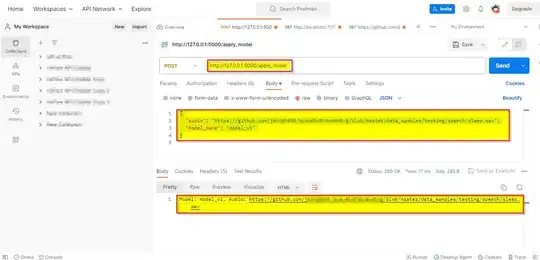
My init.py that runs the function trigger:-
import logging
import azure.functions as func
import requests
# Function to apply the specified model on the audio
def apply_model_on_audio(audio_data, model_name):
# Your code to apply the model on the audio goes here
# Example: Assuming you're using a POST request to a local API endpoint for applying the model
model_api_url = f"http://127.0.0.1:5000/apply_model"
headers = {"Content-Type": "application/json"}
data = {
"audio": audio_data,
"model_name": model_name
}
response = requests.post(model_api_url, json=data, headers=headers)
processed_audio = response.content
return processed_audio
def download_audio_file(audio_url):
response = requests.get(audio_url)
audio_data = response.content
return audio_data
# Function to upload the result to the server and get the file URL
def upload_result(result_data):
# Your code to upload the result to the server and get the file URL goes here
# Example: Assuming you're using a POST request to a local API endpoint for uploading the result and getting the file URL
upload_url = "http://localhost:8000/upload"
headers = {"Content-Type": "application/json"}
data = {
"result": result_data
}
response = requests.post(upload_url, json=data, headers=headers)
file_url = response.json().get("file_url")
return file_url
def main(req: func.HttpRequest) -> func.HttpResponse:
logging.info('Python HTTP trigger function processed a request.')
try:
req_body = req.get_json()
model_name = req_body.get('model_name')
audio_url = req_body.get('audio_url')
audio_data = download_audio_file(audio_url)
processed_audio = apply_model_on_audio(audio_data, model_name)
file_url = upload_result(processed_audio)
return func.HttpResponse(f"Processed audio file URL: {file_url}", status_code=200)
except Exception as e:
logging.error(str(e))
return func.HttpResponse("Internal Server Error", status_code=500)
Output:-
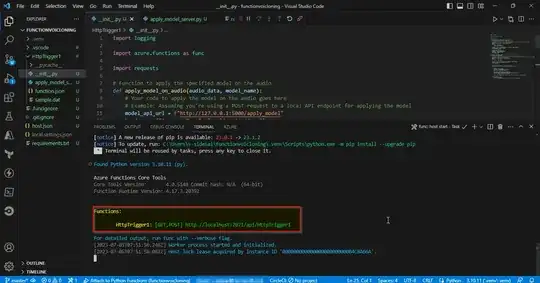
Both my server and Http Function trigger is running separately in different ports and addresses. Make sure you kill the on running server in the port or close the terminal and run the server code again.