This is what you could do in Flutter.
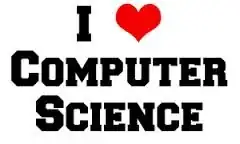
main.dart
import 'package:flutter/material.dart';
import 'home_page.dart';
void main() {
runApp(const MainApp());
}
class MainApp extends StatelessWidget {
const MainApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
home: HomePage(),
);
}
}
home_page.dart
import 'package:flutter/material.dart';
import 'util.dart';
class HomePage extends StatefulWidget {
const HomePage({super.key});
@override
State<HomePage> createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
final TextEditingController _heightController = TextEditingController();
final TextEditingController _weightController = TextEditingController();
int? _bmi;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(),
body: Padding(
padding: const EdgeInsets.symmetric(
horizontal: 20,
),
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
crossAxisAlignment: CrossAxisAlignment.center,
children: [
TextFormField(
controller: _heightController,
decoration:
const InputDecoration(helperText: "Enter Height in cm"),
),
TextFormField(
controller: _weightController,
decoration:
const InputDecoration(helperText: "Enter Weight in KG"),
),
ElevatedButton(
onPressed: () {
setState(() {
_bmi = calculatePressed(
_heightController.text, _weightController.text);
});
},
child: const Text("Calculate BMI"),
),
(_bmi != null)
? Text(
"Your Bmi is \n\n $_bmi",
)
: const SizedBox(
height: 0,
),
],
),
),
);
}
}
utils.dart
calculatePressed(String height, String wt) {
var tall = height.toString();
var weight = wt.toString();
var cTall = int.parse(tall);
var dWeight = int.parse(weight);
var dTall = cTall / 100;
var bmi = (dWeight / dTall * dTall).toInt();
// var result = 'Your Bmi is \n\n $bmi';
return bmi;
}