Ugly images below, but hopefully also an instructive example:
% Create dummy image
x = 0:4:255;
origimage = uint8(repmat(x,64,1));
origimage(:,:,2) = 100;
origimage(:,:,3) = 100;
% create dummy mask
colormask = logical(zeros(64,64));
colormask(1:20,30:50) = 1;
% display original image and mask
figure(1);image(origimage);
figure(2); imagesc(colormask);
% create altered image
newimage = origimage;
newimage(colormask) = 255; %changes first channel to 255 in masked pixels
figure(3); image(newimage);
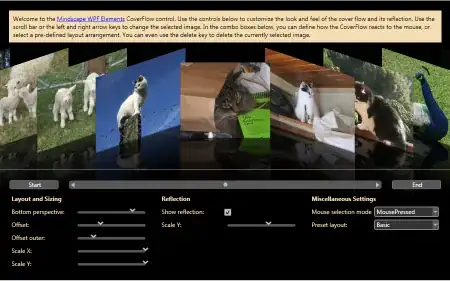
Since colormask is a logical, you can use it directly to select the relevant pixels in the original image and modify them in one command. Hope this helps.
**Added 7/12/2023 in response to comment about changing other channels:
You have flexibility to change the other channels. My original code only changed the red channel because my mask was only 2D.
% change all three channels together
colormask2 = logical(zeros(64,64,3));
colormask2(1:20,30:50,1:3) = 1;
newimage2 = origimage;
newimage2(colormask2) = 200;
Alternate and more flexible approach:
%create 3D mask with different numbers for each channel
colormask3 = uint8(zeros(64,64,3));
colormask3(1:20,30:50,1) = 1;
colormask3(1:20,30:50,2) = 2;
colormask3(1:20,30:50,3) = 3;
newimage4 = origimage;
newimage4(logical(colormask3)) = 200; %gives same image as prior example
figure(4); image(newimage4);
newimage5 = origimage;
newimage5 = newimage5 + 10*colormask3; %adds 10 to red, 20 to green, 30 to blue
figure(5); image(newimage5);
% add to the original image itself *1/2 for red, *1 for green, and *1.5
% for blue
newimage6 = origimage + origimage.*colormask3/2;
figure(6); image(newimage6);