Below is some example code showing one way to pass data from the presented view controller (VC2) to the presenting view controller (VC1).
VC2 defines var callback: (() -> Void)?
, which is a function type. It defines a function that has no parameters and returns nothing. Prepare(for segue:) in VC1 provides the callback function to VC2 as a block of code. The callback updates VC1's data and reloads VC1's table view.
In VC2, when Done is selected, it calls the callback function before being dismissed.
class VC1: UIViewController, UITableViewDataSource {
var data = [1, 2, 3, 4, 5, 6, 7]
@IBOutlet weak var tableView: UITableView!
override func viewDidLoad() {
super.viewDidLoad()
tableView.dataSource = self
}
// MARK: - TableViewDataSource
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return data.count
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCell(withIdentifier: "Cell", for: indexPath)
cell.textLabel?.text = "\(data[indexPath.row])"
return cell
}
// MARK: - Navigation
override func prepare(for segue: UIStoryboardSegue, sender: Any?) {
if segue.identifier == "Show Number" {
if let cell = sender as? UITableViewCell {
let indexPath = tableView.indexPath(for: cell)
if let vc2 = segue.destination as? VC2 {
vc2.number = data[indexPath!.row]
vc2.callback = {
self.data[indexPath!.row] = vc2.number
self.tableView.reloadData()
}
}
}
}
}
}
class VC2: UIViewController {
var number = 0
var callback: (() -> Void)?
@IBOutlet weak var numberLabel: UILabel!
override func viewDidLoad() {
super.viewDidLoad()
numberLabel.text = "\(number)"
}
@IBAction func add100Selected(_ sender: UIButton) {
number += 100
numberLabel.text = "\(number)"
}
@IBAction func doneSelected(_ sender: UIButton) {
callback?()
dismiss(animated: true)
}
}
Full Screen Modal Segway
Using a Full Screen modal segue, you are forced to use the Done button, which always saves the changes.
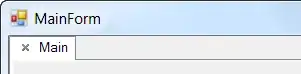
Page Sheet Modal Segway (swipeable)
Using a Page Sheet modal segue, the changes are not saved if you swipe VC2 away. If you want the changes to be saved no matter how you dismiss VC2, you would have to call the callback function right after you modify the data (at the end of add100Selected, in my example).
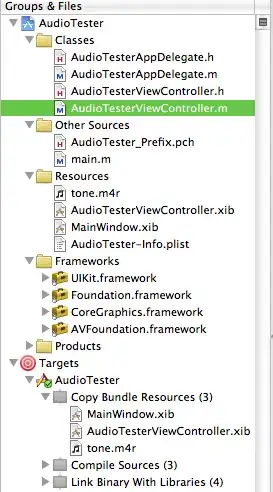
I used the same VC code for each video (just changed the segue presentation in Interface Builder).
Interface Builder
Here's how I set up the two VC's in Interface Builder. I selected the segue in the image, so you can see its settings in the Attributes inspector.
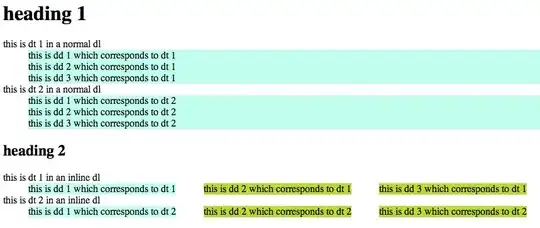