Try following :
static void Main(string[] args)
{
DataTable dt = new DataTable();
dt.Columns.Add("Personid", typeof(int));
dt.Columns.Add("OrginalDate", typeof(int));
dt.Columns.Add("ConvertedDate", typeof(DateTime));
dt.ColumnsAdd("OriginalTime", typeof(int));
dt.Columns.Add("ConvertedTime", typeof(TimeSpan));
dt.Rows.Add(new object[] { 115, 45099, DateTime.Parse("6/22/2023 12:00:00"), 1206, TimeSpan.Parse("12:06") });
dt.Rows.Add(new object[] { 115, 45099, DateTime.Parse("6/22/2023 12:00:00"), 1859, TimeSpan.Parse("18:59") });
dt.Rows.Add(new object[] { 631, 45099, DateTime.Parse("6/22/2023 12:00:00"), 1449, TimeSpan.Parse("14:49") });
dt.Rows.Add(new object[] { 631, 45099, DateTime.Parse("6/22/2023 12:00:00"), 1722, TimeSpan.Parse("17:22") });
dt.Rows.Add(new object[] { 631, 45099, DateTime.Parse("6/22/2023 12:00:00"), 1819, TimeSpan.Parse("18:19") });
dt.Rows.Add(new object[] { 631, 45099, DateTime.Parse("6/22/2023 12:00:00"), 2006, TimeSpan.Parse("20:06") });
var groups = dt.AsEnumerable().GroupBy(x => x.Field<int>("Personid"));
var maxItems = groups.Max(x => x.Count()) / 2;
DataTable pivot = new DataTable();
pivot.Columns.Add("Personid", typeof(int));
pivot.Columns.Add("OrginalDate", typeof(int));
pivot.Columns.Add("ConvertedDate", typeof(DateTime));
for (int i = 1; i <= maxItems; i++)
{
pivot.Columns.Add("EnterTime" + i, typeof(TimeSpan));
pivot.Columns.Add("ExitTime" + i, typeof(TimeSpan));
}
foreach (var group in groups)
{
DataRow newRow = pivot.Rows.Add();
newRow["Personid"] = group.First()["Personid"];
newRow["OrginalDate"] = group.First()["OrginalDate"];
newRow["ConvertedDate"] = group.First()["ConvertedDate"];
for(int i = 0; i < group.Count() / 2; i++)
{
newRow["EnterTime" + (i + 1)] = group.Skip(2 * i).First().Field<TimeSpan>("ConvertedTime");
newRow["ExitTime" + (i + 1)] = group.Skip((2 *i) + 1).First().Field<TimeSpan>("ConvertedTime");
}
}
}
Results
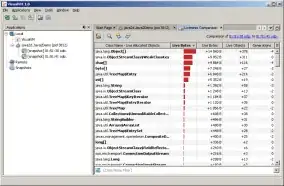