If you want to use Windows.Media.SpeechRecognition.SpeechRecognizer
in .NET project, you can add Windows.winmd and System.Runtime.WindowsRuntime.dll in the project References.
Here is my file location for your reference.
C:\Program Files (x86)\Windows Kits\10\UnionMetadata\10.0.16299.0\Windows.winmd
C:\Program Files (x86)\Reference Assemblies\Microsoft\Framework.NETCore\v4.5\ystem.Runtime.WindowsRuntime.dll
Update
My console code sample
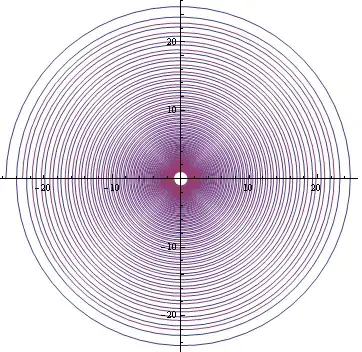
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Windows.Media.SpeechRecognition;
namespace ConsoleApp1
{
internal class Program
{
static async Task Main(string[] args)
{
var recognizer = new SpeechRecognizer();
await recognizer.CompileConstraintsAsync();
recognizer.Timeouts.InitialSilenceTimeout = TimeSpan.FromSeconds(5);
recognizer.Timeouts.EndSilenceTimeout = TimeSpan.FromSeconds(20);
recognizer.UIOptions.AudiblePrompt = "Say whatever you like, I'm listening";
recognizer.UIOptions.ExampleText = "This a sample Test";
recognizer.UIOptions.ShowConfirmation = true;
recognizer.UIOptions.IsReadBackEnabled = true;
recognizer.Timeouts.BabbleTimeout = TimeSpan.FromSeconds(5);
var result = await recognizer.RecognizeWithUIAsync();
if (result != null)
{
StringBuilder builder = new StringBuilder();
builder.AppendLine($"I have {result.Confidence} confidence that you said [ {result.Text}] " + $"and it took {result.PhraseDuration.TotalSeconds} seconds to say it " + $"starting at {result.PhraseStartTime:g}");
var alternates = result.GetAlternates(10);
builder.AppendLine($"There were {alternates?.Count} alternates - listed below (if any)");
if (alternates != null)
{
foreach (var alternate in alternates)
{
builder.AppendLine($"Alternate {alternate.Confidence} confident you said [ {alternate.Text}]");
}
}
}
}
}
}