Currently, error message is "azure.common.AzureMissingResourceHttpError: The specified parent path does not exist. ErrorCode: ParentNotFound"
The above error when you don't specified path or directory in your storage account.
Here is my structure in the storage account :
Storage account name = venkat123
fileshare=fileshare1
directory=directory1
filename=day.csv
Portal:
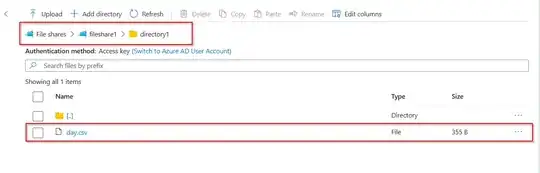
You can use the below code to read the CSV file with the account URL and key.
For that, you need to install the package of azure-storage-file-share.
Code:
from azure.storage.fileshare import ShareServiceClient, ShareClient, ShareDirectoryClient, ShareFileClient
import pandas as pd
import io
account_url = "https://venkat123.file.core.windows.net"
account_key = "xxxxxx"
service_client = ShareServiceClient(account_url=account_url, credential=account_key)
share_name = "fileshare1"
share_client = service_client.get_share_client(share_name)
# Get a ShareDirectoryClient object to connect to the directory
directory_path = "directory1"
directory_client = share_client.get_directory_client(directory_path)
file_name = "day.csv"
file_client = directory_client.get_file_client(file_name)
file_contents = file_client.download_file().readall()
csv_data = file_contents.decode('utf-8')
df = pd.read_csv(io.StringIO(csv_data))
print(df)
Output:
Numeric Numeric-2 Numeric-Suffix
0 1 1 1st
1 2 2 2nd
2 3 3 3rd
3 4 4 4th
4 5 5 5th
5 6 6 6th
6 7 7 7th
7 8 8 8th
8 9 9 9th
9 10 10 10th
10 11 11 11th
11 12 12 12th
12 13 13 13th
13 14 14 14th
14 15 15 15th
15 16 16 16th
16 17 17 17th
17 18 18 18th
18 19 19 19th
19 20 20 20th
20 21 21 21st
21 22 22 22nd
22 23 23 23rd
23 24 24 24th
24 25 25 25th
25 26 26 26th
26 27 27 27th
27 28 28 28th
28 29 29 29th
29 30 30 30th
30 31 31 31st

Reference:
Azure Storage File Share client library for Python | Microsoft Learn