I created an Azure AD Application and added App role like below:
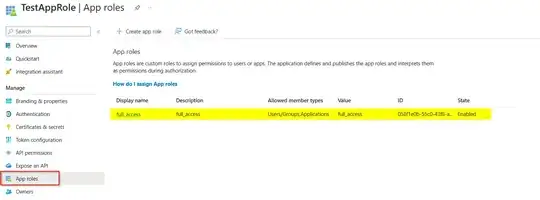
Now, I created an Azure AD Group and added Service Principal as member:
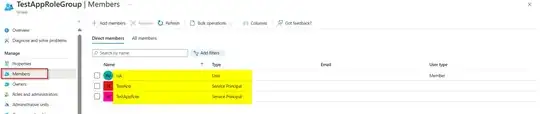
Assigned App role to the Azure AD Group:
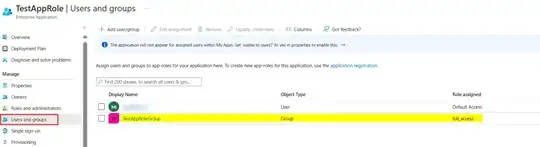
My questions is: How do I verify that the service principal has the correct role due to its group membership? Is there some workaround I can use?
You can make use of below code to fetch the app roles assigned to the Service Principal:
using Azure.Identity;
using Azure.Core;
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Text.Json;
using System.Net.Http;
using System.Threading.Tasks;
using Newtonsoft.Json;
using Newtonsoft.Json.Linq;
class Program
{
static async Task Main(string[] args)
{
string accessToken = "AccessToken";
string servicePrincipalId = "ServicePrincipalObjID";
var appRoles = await GetAppRolesAsync(accessToken, servicePrincipalId);
foreach (var appRole in appRoles)
{
Console.WriteLine($"App role: " + $"{"Displayname:" + appRole.DisplayName}");
Console.WriteLine($" {"Value:" + appRole.Value}");
Console.WriteLine ($" {"Id:" + appRole.Id}" );
}
}
public static async Task<List<AppRole>> GetAppRolesAsync(string accessToken, string servicePrincipalId)
{
using (var client = new HttpClient())
{
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Bearer", accessToken);
var response = await client.GetAsync($"https://graph.microsoft.com/v1.0/servicePrincipals/{servicePrincipalId}/appRoles");
if (!response.IsSuccessStatusCode)
{
throw new Exception($"Failed to get app roles: {response.StatusCode}");
}
var json = await response.Content.ReadAsStringAsync();
var jsonObject = JObject.Parse(json);
var jsonArray = jsonObject["value"].ToString();
var appRoles = JsonConvert.DeserializeObject<List<AppRole>>(jsonArray);
return appRoles;
}
}
public class AppRole
{
[JsonProperty("id")]
public string Id { get; set; }
[JsonProperty("allowedMemberTypes")]
public string[] AllowedMemberTypes { get; set; }
[JsonProperty("description")]
public string Description { get; set; }
[JsonProperty("displayName")]
public string DisplayName { get; set; }
[JsonProperty("isEnabled")]
public bool IsEnabled { get; set; }
[JsonProperty("value")]
public string Value { get; set; }
}
}
The App role assigned to the Azure AD Application with the ID displayed successfully like below:
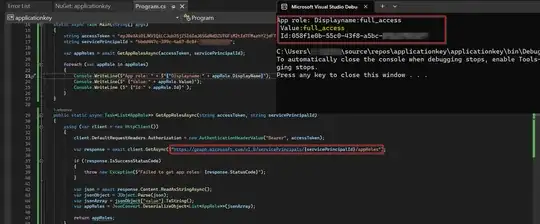
App role: Displayname:full_access
Value:full_access
Id: xxxxxx