A simple one-liner method is to subsample as follows: img_smaller = img[::10, ::10, :]
. This will subsample it down 10x, but the image will look coarse as no interpolation was applied, and edges may look clipped if the dimensions aren't multiples of 10:
Before downsampling:
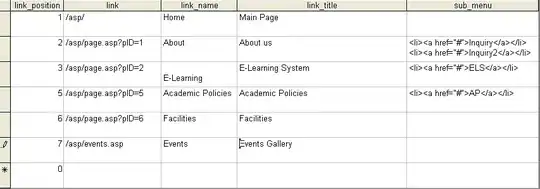
Downsampling using array slicing:
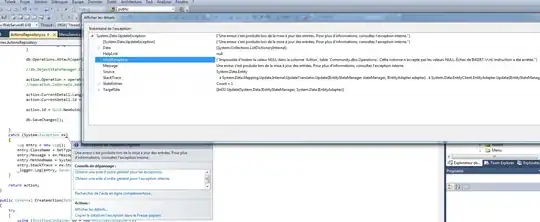
Another approach is to downsample the image after loading it using PIL
. After setting downsample=12
, i.e. 12x downsampling:
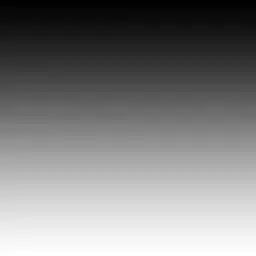
PIL
downsampling:
from PIL import Image
#Load image, or make a test image
np.random.seed(0)
img = Image.fromarray( np.random.randint(0, 255, size=(100, 100, 3), dtype=np.ubyte))
img = Image.open('../image.png')
#Subsample 12x and apply interpolation
downsample = 12
img_small = img.resize((img.height // downsample, img.width // downsample),
resample=Image.BICUBIC)
h, w = img_small.height, img_small.width
img_small_arr = np.asarray(img_small)
#Plot
ax = plt.figure(figsize=(3, 3)).add_subplot(projection='3d')
xx, yy = np.meshgrid(np.linspace(0, 1, w), np.linspace(0, 1, h))
zz = np.ones((h, w))
ax.plot_surface(xx, yy, zz, facecolors=img_small_arr / 255, shade=False)
#facecolor=[0,0,0,0], linewidth=1) #optionally add a 'grid' effect
One-liner method using slicing:
#Downsample using slicing
img_small_arr = np.asarray(img)[::10, ::10, :]
#New dimensions
h, w, _ = img_small_arr.shape
#Rest of the plotting, as before
ax = plt.figure(figsize=(3, 3)).add_subplot(projection='3d')
xx, yy = np.meshgrid(np.linspace(0, 1, w), np.linspace(0, 1, h))
zz = np.ones((h, w))
ax.plot_surface(xx, yy, zz, facecolors=img_small_arr / 255, shade=False)