If you have Microsoft 365:
=SUBSTITUTE(
TEXTJOIN(
" ",
,
LET(
a, SUBSTITUTE(A1, CHAR(10), "~!"),
b, TEXTSPLIT(a, , {" ", "~"}),
c, ISNUMBER(FIND({"$", "."}, b)),
d, BYROW(c, LAMBDA(arr, OR(arr))),
e, FILTER(b, NOT(d)),
e
)
),
"!",
CHAR(10)
)
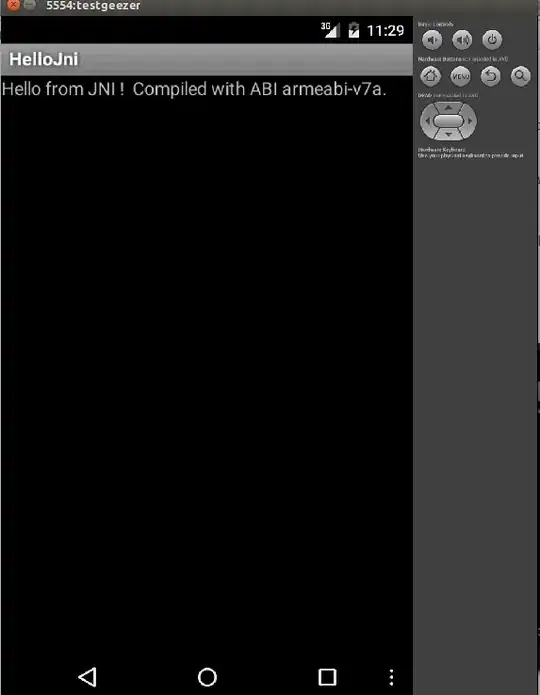
Algorithm
- Replace the line feed with
~!
- Enables splitting on
space
and tilde
, leaving the !
to replace the linefeeds when we rejoin the string
- Filter the resultant array removing substrings that contain
$
or .
- Note that when
find_text
is an array, the result will be a multi-column array with one column for each element
- We use the
BYROW
function to reduce this to a single column array for use in the FILTER
function.
- Rejoin with spaces
- Then replace the
!
with linefeeds
If you do not have Microsoft 365, this can also be accomplished using Power Query, available in Windows Excel 2010+ and Excel 365 (Windows or Mac)
To use Power Query
- Select some cell in your Data Table
Data => Get&Transform => from Table/Range
or from within sheet
- When the PQ Editor opens:
Home => Advanced Editor
- Make note of the Table Name in Line 2
- Paste the M Code below in place of what you see
- Change the Table name in line 2 back to what was generated originally.
- Read the code and comments. The algorithm is very similar to the formula above
let
//Change next line to reflect your actual data source
Source = Excel.CurrentWorkbook(){[Name="Table3"]}[Content],
#"Changed Type" = Table.TransformColumnTypes(Source,{{"Column1", type text}}),
#"Remove Currency" = Table.TransformColumns(#"Changed Type",
//split each cell on the line feed
// then split each split on space
// remove items that contain $ or .
// reassemble
{"Column1", (c)=>
try
let
lines = Text.Split(c,"#(lf)"),
removeCurr = List.Transform(lines, (d)=>
let
words = Text.Split(d," "),
notCurr = List.Select(words, each not Text.Contains(_,"$") and not Text.Contains(_,"."))
in
notCurr)
in
Text.Combine(List.Transform(removeCurr, each Text.Combine(_," ")), "#(lf)")
otherwise null}
)
in
#"Remove Currency"