When I download a file using FileService or ShareFileClient (azure-storage or azure-storage-file libraries) it changes the creation date and modified date, so it downloads a new file with metadata changed.
The current updated time of the file in the file share is not changed by the download_file()
function. After downloading the file, the file's current modified time should remain the same.
It's possible that the file's last modified time in the file share was changed for another reason if you monitor a different last modified time after downloading the file.
You can use the below code to check the downloading file from file share both creation time and modified time.
Code:
from azure.storage.fileshare import ShareServiceClient
connection_string = "your-connection-string"
share_name = "fileshare1"
file_path = "directory1/Icon-60@3x.png"
local_file_path = "./test.png"
# Initialize the ShareServiceClient
service_client = ShareServiceClient.from_connection_string(connection_string)
# Get a reference to the file
share_client = service_client.get_share_client(share_name)
file_client = share_client.get_file_client(file_path)
original_creation_time=file_client.get_file_properties().creation_time
#Get the last modified time of the file before downloading it
original_last_modified = file_client.get_file_properties().last_modified
# Download the file
with open(local_file_path, "wb") as local_file:
downloaded_file = file_client.download_file()
local_file.write(downloaded_file.readall())
# Get the last modified time of the file after downloading it
new_last_modified = file_client.get_file_properties().last_modified
#print(new_last_modified)
new_creation_time=file_client.get_file_properties().creation_time
#print(new_creation_time)
metadata=file_client.get_file_properties().metadata
print(metadata)
# Compare the last modified times
if original_last_modified == new_last_modified:
print("Last modified time was not changed.")
else:
print("Last modified time was changed.")
if original_creation_time==new_creation_time:
print("Creation time Was not changed.")
else:
print("Creation time was Changed.")
Output:
{'test': 'demo'}
Last modified time was not changed.
Creation time Was not changed.
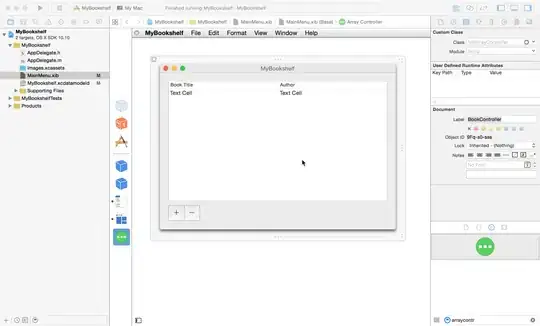
Portal:
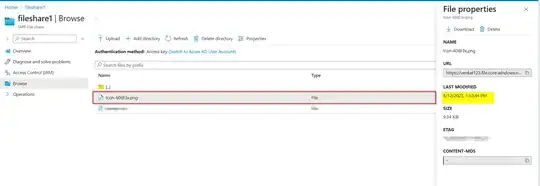
Reference:
Azure Storage File Share client library for Python | Microsoft Learn