Oh I think I see what to do:
https://www.tiktok.com/@virtual.tails/video/7256528174178127147?_r=1&_t=8eoEPjc11oJ
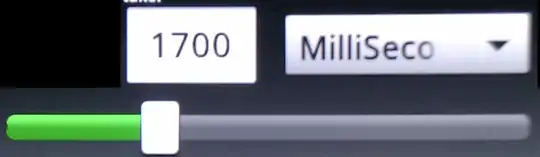
You have to really make a huge black background for each letter not just a tiny drop shadow.
for i := 0; i < 100; i++ {
angle := float64(i) / float64(smoothness) * 2 * math.Pi
xOffset := math.Cos(angle) * 4
yOffset := math.Sin(angle) * 4
dc.DrawStringAnchored(letter, x+xOffset, y+yOffset, 0.5, 0.5)
}
Update: it's easier to just do this in python:
https://stackoverflow.com/a/62941753/148736
pip3 install pillow
from PIL import Image, ImageDraw, ImageFont, ImageFilter, ImageChops
import sys
import random
filename = sys.argv[1]
x = int(sys.argv[2])
y = int(sys.argv[3])
text = sys.argv[4]
result = sys.argv[5]
image = Image.open(filename)
bg = ImageDraw.Draw(image)
font = ImageFont.truetype('font.ttf',96)
colors = ['white', 'pink', 'green', 'orange', 'red', 'yellow', 'purple', 'cyan']
fill_color = random.choice(colors)
ranX = random.randint(-10, 10)
ranY = random.randint(-10, 10)
bg.text((x + ranX, y + ranY), text, fill=fill_color, font=font, spacing = 4, align = 'center', stroke_width=8, stroke_fill='black')
image.save(result)