Based on the reference for the css hue-rotate
algorithm at https://drafts.fxtf.org/filter-effects/#feColorMatrixElement and some other references on this site at ...
... a function for applying hue-rotate
in PowerShell would be something like this:
function Invoke-HueRotate
{
param( [uint] $Color, [decimal] $Degrees )
# split color into r, g and b channels
$r = ($Color -shr 16) -band 0xFF;
$g = ($Color -shr 8) -band 0xFF;
$b = ($Color -shr 0) -band 0xFF;
# convert degrees to radians and evaluate cos / sin
$rad = $Degrees * [Math]::PI / 180;
$cos = [Math]::Cos($rad);
$sin = [Math]::Sin($rad);
# long-hand matrix definition
# see https://drafts.fxtf.org/filter-effects/#feColorMatrixElement
# where it says 'For type="hueRotate"'
$a00 = 0.213 + ( 0.787 * $cos) + (-0.213 * $sin);
$a01 = 0.715 + (-0.715 * $cos) + (-0.715 * $sin);
$a02 = 0.072 + (-0.072 * $cos) + ( 0.928 * $sin);
$a10 = 0.213 + (-0.213 * $cos) + ( 0.143 * $sin);
$a11 = 0.715 + ( 0.285 * $cos) + ( 0.140 * $sin);
$a12 = 0.072 + (-0.072 * $cos) + (-0.283 * $sin);
$a20 = 0.213 + (-0.213 * $cos) + (-0.787 * $sin);
$a21 = 0.715 + (-0.715 * $cos) + ( 0.715 * $sin);
$a22 = 0.072 + ( 0.928 * $cos) + ( 0.072 * $sin);
# long-hand matrix multiplication
$r2 = ($a00 * $r) + ($a01 * $g) + ($a02 * $b);
$g2 = ($a10 * $r) + ($a11 * $g) + ($a12 * $b);
$b2 = ($a20 * $r) + ($a21 * $g) + ($a22 * $b);
# re-combine r, g and b channels
$result = [uint](
([Math]::Clamp([int]$r2, 0, 255) -shl 16) `
-bor
([Math]::Clamp([int]$g2, 0, 255) -shl 8) `
-bor
([Math]::Clamp([int]$b2, 0, 255) -shl 0) `
);
return $result;
}
(I've avoided any matrix libraries to keep it self-contained, but you could simplify it down if you don't mind taking a dependency on something that can do the math cleaner.)
If you add the following utility methods as well:
function Invoke-InvertColor
{
param( [uint] $Color )
return $Color -bxor 0xFFFFFF;
}
function ConvertFrom-HtmlColor
{
param( [string] $Value )
return [System.Convert]::ToUInt32($Value.Substring(1), 16);
}
function ConvertTo-HtmlColor
{
param( [uint] $Value )
return "#" + $Value.ToString("X2").PadLeft(6, "0");
}
you could use it as follows:
$color = "#CC1100";
$original = ConvertFrom-HtmlColor -Value $color;
ConvertTo-HtmlColor $original;
#CC1100
$inverted = Invoke-InvertColor -Color $original;
ConvertTo-HtmlColor $inverted;
#33EEFF
$rotated = Invoke-HueRotate -Color $inverted -Degrees 180;
ConvertTo-HtmlColor $rotated;
#FFA190
Note that this gives a different result to your example for
Invert Hue-rotate 180∘
#CC1100 -> #33EEFF -> #FF4433
but the result seems to tally up with the formulas in the first link in this answer, and gets the same result as the implementations in the other links, so you might need to elaborate on what algorithm / implementation you're using for hue-rotate
if you're after a different result...
Update
Additional verification of the function result:
<style>
div {
background-color: #33EEFF;
width: 250px;
height: 250px;
filter: hue-rotate(180deg);
}
</style>
<div>
</div>
gives this:
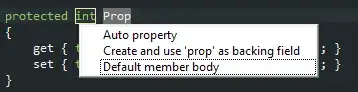
and if you use the color dropper in your favourite image editor it'll tell you the peach color is #FFA190
which matches the result from the PowerShell code above...