I have a .NET 6 console app that runs on multiple servers to monitor apps on them.
The multiple servers already have their environment variable setup for other apps that use these values.
For example:
Server A -> DOTNET_ENVIRONMENT = dev
Server B -> DOTNET_ENVIRONMENT = qa
Server C -> DOTNET_ENVIRONMENT = prod
Server D -> DOTNET_ENVIRONMENT = prod
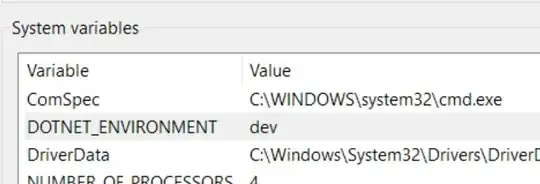
Even though both servers C and D are prod servers, they need different appsettings
because they monitor different apps in those servers. So, having one appsettings.prod.json
to be used in servers C and D won't work in this case.
I don't want to mess with the value of DOTNET_ENVIRONMENT
either because other apps running on that server are using it.
What I've done so far
I named appsettings.json
files of my console app based on the server's name.
For example:
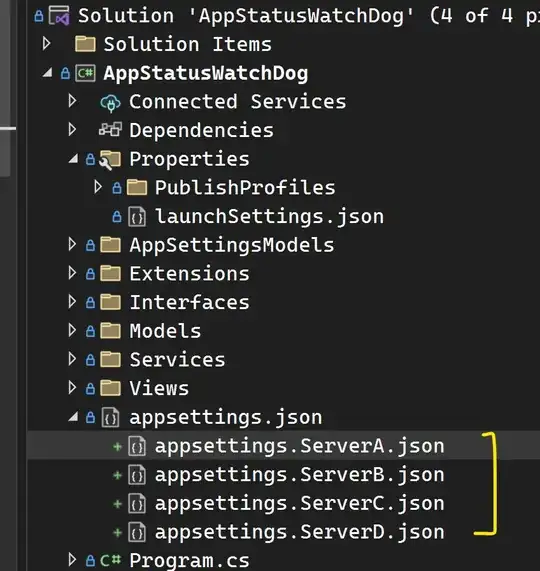
Added launchsettings
profile to pass machine name as environment variable (for my testing):
{
"profiles": {
"AppStatusWatchDog (ServerA)": {
"commandName": "Project",
"environmentVariables": {
"MachineName": "ServerA"
},
"dotnetRunMessages": "true"
}
}
}
And tried to load correct appsettings.json
during startup:
public static void Main(string[] args)
{
var pathToExe = Process.GetCurrentProcess().MainModule.FileName;
var pathToContentRoot = Path.GetDirectoryName(pathToExe);
// Just calling Environment.MachineName is adequate here. GetEnvironmentVariable("MachineName") is here because we supply MachineName's value during local testing using launch profile.
var machineName = Environment.GetEnvironmentVariable("MachineName") ?? Environment.MachineName;
var config = new ConfigurationBuilder()
.SetBasePath(pathToContentRoot)
.AddJsonFile("appsettings.json", optional: false, reloadOnChange: true)
.AddJsonFile($"appsettings.{machineName}.json", optional: true, reloadOnChange: true)
.AddEnvironmentVariables()
.Build();
// Rest of the code here...
}
Here config
loads all the settings I have, i.e. appsettings.json
, appsettings.ServerA.json
and the environment variables which is good .
The issue comes up when I try to use config by injecting IConfiguration
into a constructor of some class at which point, it loads appsettings.json
and the environment variables but never the appsettings.ServerA.json
.
What am I doing wrong here?