Edit
If you wish to turn this into a Modifier you can do it as
fun Modifier.drawImageSection(
rect: Rect,
painter: Painter
) = this.then(
Modifier.drawWithCache {
val painterSize = painter.intrinsicSize
if (painterSize != androidx.compose.ui.geometry.Size.Unspecified){
val topLeft = Offset(
x = rect.topLeft.x.coerceIn(0f, painterSize.width),
y = rect.topLeft.y.coerceIn(0f, painterSize.height)
)
val updatedRect = Rect(
offset = topLeft,
size = androidx.compose.ui.geometry.Size(
width = (rect.width ).coerceIn(0f, painterSize.width- topLeft.x),
height = (rect.height ).coerceIn(0f, painterSize.height- topLeft.y)
),
)
val scaleX = size.width / painterSize.width
val scaleY = size.height / painterSize.height
// We scale from Bitmap dimension to Composable dimension
val left = updatedRect.left * scaleX
val top = updatedRect.top * scaleY
val right = updatedRect.right * scaleX
val bottom = updatedRect.bottom * scaleY
onDrawWithContent {
clipRect(
left = left,
top = top,
right = right,
bottom = bottom
) {
with(painter) {
draw(size)
}
}
}
}else {
onDrawWithContent {}
}
}
)
Usage
Box(
modifier = Modifier
.border(2.dp, Color.Green)
.size(200.dp)
.drawImageSection(
rect = Rect(
offset = Offset(170f,18f),
size = androidx.compose.ui.geometry.Size(205f, 358f)
),
painter = painter
)
)
Result
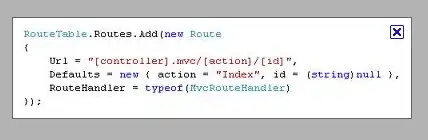
Because it's a picture of original image with incorrect size you won't be able to get correct results.
The image you posted is 417x419px, results i get measuring with a tool for the image you shared are
// These are the dimensions on real image you want to clip at
val left = 170f
val top = 18f
val right = (170f + 205f)
val bottom = (18f + 358f)
Using this information first you need to get dimensions of image. It can be
retrieved using painter.intrinsicSize.
Then you need to get scale for width and height for Compsosable dimensions(200.dp in pixel) versus image dimensions.
After getting these, since scaling you can clip inside a rect
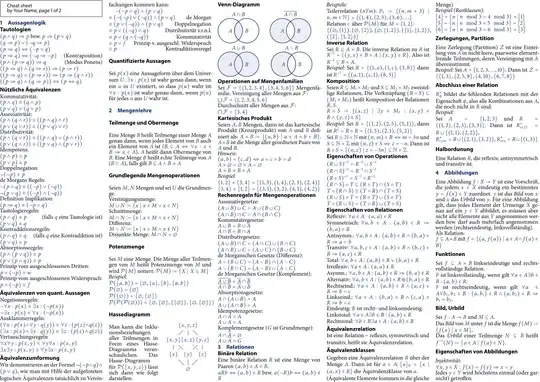
val painter = rememberAsyncImagePainter(
model = ImageRequest.Builder(LocalContext.current)
.data(R.drawable.test)
.size(Size.ORIGINAL) // Set the target size to load the image at.
.build()
)
Column(
modifier = Modifier
.fillMaxSize()
.padding(20.dp)
) {
Text(text = "painter size: ${painter.intrinsicSize}")
Image(
modifier = Modifier
.border(2.dp, Color.Red)
.drawWithContent {
val painterSize = painter.intrinsicSize
if (painterSize != androidx.compose.ui.geometry.Size.Unspecified &&
painterSize.width != 0f &&
painterSize.height != 0f
) {
val scaleX = size.width / painterSize.width
val scaleY = size.height / painterSize.height
// We scale from Bitmap dimension to Composable dimension
val left = 170f * scaleX
val top = 18f * scaleY
val right = (170f + 205f) * scaleX
val bottom = (18f + 358f) * scaleY
clipRect(
left = left,
top = top,
right = right,
bottom = bottom
) {
this@drawWithContent.drawContent()
}
}
},
painter = painter,
contentDescription = null,
contentScale = ContentScale.FillBounds
)
}
}