It may be possible, but I think an easier approach would be to use custom annotations, instead of making the ticks do two separate things at once.
In this modified code, I store the location and text for the dates you want in red. Then, I loop through these locations and text values and annotate them onto the figure, using ax.annotate
which I think is a better fit for what you are trying to accomplish:
import pandas as pd
import matplotlib.pyplot as plt
def annotations(time_range):
data = []
annotations = []
for i in time_range:
# If we want a custom tick, store it's data point and annotation
if (i.strftime("%H:%M:%S") == "00:00:00"):
data.append(i)
annotations.append(i.strftime("%Y-%m-%d"))
return data, annotations
fig, ax = plt.subplots(figsize=(20, 4))
time_range = pd.date_range('01.01.2020 00:00:00', '01.03.2020 01:00:00', freq='2H')
ax.plot(time_range, len(time_range)*[1])
labels = [i.strftime("%H:%M") for i in time_range]
ax.set_xticks(time_range, labels)
location, annnotations = annotations(time_range)
for x_loc, ann in zip(location, annnotations):
y_loc = .93
ax.annotate(
text=ann, # The text we are appending to the figure
xy=(x_loc, y_loc), # The (x,y) coordinates of that text in the axes DATA coordinates
annotation_clip=False, # Tell MPL to not clip annotations outside of the axes range
color='red',
fontsize=14,
ha='center',
va='center'
)
This produces what you want, and I think is clearer than trying to do some intense tick label customization. Notice that I hard-coded y_loc
to be 0.93, which corresponds to the value of .93 on your y-axis where I wanted the annotations to be. You could probably automate this by getting the lower y limit of your axis and subtracting some constant value, but I did not do this for simplicity.
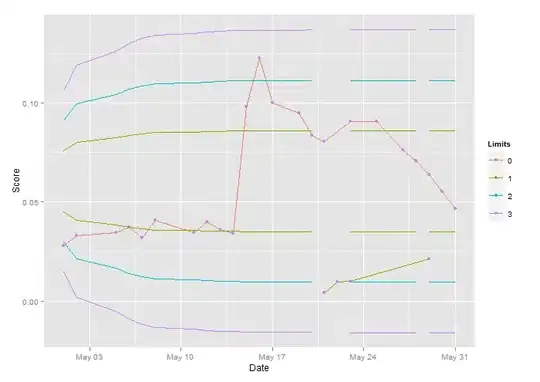