You can make use of Rest API to list all your custom domains and use another API's related to creating and updating Custom domains with host binding in and call it with GET, PUT or POST request in your C# code like below:-
My C# Http Trigger function code:-
Rest API reference:-
Reference :- My SO thread answer
using Azure.Core;
using Azure.Identity;
using Newtonsoft.Json;
using System;
using System.Net.Http;
using System.Threading.Tasks;
using Microsoft.AspNetCore.Http;
using Microsoft.AspNetCore.Mvc;
using Microsoft.Azure.WebJobs;
using Microsoft.Azure.WebJobs.Extensions.Http;
using Microsoft.Extensions.Logging;
using System.Net.Http.Json;
namespace FunctionApp1
{
public static class Function1
{
[FunctionName("Function1")]
public static async Task<IActionResult> Run(
[HttpTrigger(AuthorizationLevel.Function, "get", Route = null)] HttpRequest req,
ILogger log)
{
log.LogInformation("C# HTTP trigger function processed a request.");
var token = await GetAccessToken("83331f4e-7f45-4ce4-99ed-af9038592395", "c0c952e9-5254-45b5-b838-6d26a31435cb", "Cnd8Q~Ro6wHqvMGQUyvqrEgguL0nl-gYmTYkDcPI");
var results = await GetResults(token);
return new OkObjectResult(results);
}
private static async Task<string> GetAccessToken(string tenantId, string clientId, string clientKey)
{
var credentials = new ClientSecretCredential(tenantId, clientId, clientKey);
var result = await credentials.GetTokenAsync(new TokenRequestContext(new[] { "https://management.azure.com/.default"
}), default);
return result.Token;
}
private static async Task<string> GetResults(string token)
{
var httpClient = new HttpClient
{
BaseAddress = new Uri("https://management.azure.com/subscriptions/")
};
string URI = $"0151c365-f598-44d6-b4fd-e2b6e97cb2a7/providers/Microsoft.DomainRegistration/domains?api-version=2022-03-01";
httpClient.DefaultRequestHeaders.Remove("Authorization");
httpClient.DefaultRequestHeaders.Add("Authorization", "Bearer " + token);
HttpResponseMessage response = await httpClient.GetAsync(URI);
var HttpsResponse = await response.Content.ReadAsStringAsync();
//var JSONObject = JsonConvert.DeserializeObject<object>(HttpsResponse);
//return response.StatusCode.ToString();
return HttpsResponse;
}
}
}
Output:-
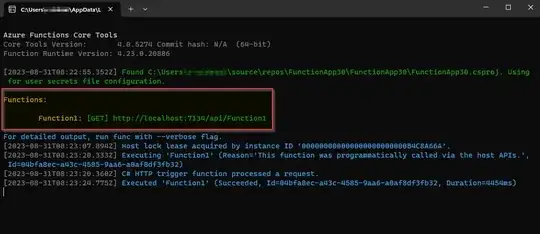
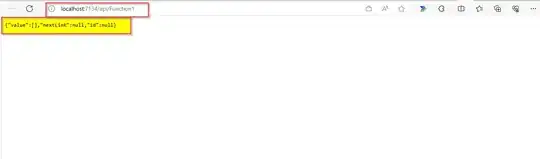
Similar you can use this API to Web Apps - Create Or Update Host Name Binding - REST API (Azure App Service) | Microsoft Learn
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Text;
using System.Threading.Tasks;
using Microsoft.AspNetCore.Http;
using Microsoft.AspNetCore.Mvc;
using Microsoft.Azure.WebJobs;
using Microsoft.Azure.WebJobs.Extensions.Http;
using Microsoft.Extensions.Logging;
using Newtonsoft.Json;
namespace FunctionApp1
{
public static class Function1
{
[FunctionName("UpdateHostNameBindingFunction")]
public static async Task<IActionResult> Run(
[HttpTrigger(AuthorizationLevel.Function, "post", Route = null)] HttpRequest req,
ILogger log)
{
log.LogInformation("C# HTTP trigger function processed a request.");
string requestBody = await new StreamReader(req.Body).ReadToEndAsync();
var requestParams = JsonConvert.DeserializeObject<HostNameBindingRequest>(requestBody);
var token = await GetAccessToken("<tenant-id>", "<client-id>", "<client-secret>");
var response = await UpdateHostNameBinding(token, requestParams);
return new OkObjectResult(response);
}
private static async Task<string> GetAccessToken(string tenantId, string clientId, string clientSecret)
{
var credentials = new ClientSecretCredential(tenantId, clientId, clientSecret);
var result = await credentials.GetTokenAsync(new TokenRequestContext(new[] { "https://management.azure.com/.default" }), default);
return result.Token;
}
private static async Task<string> UpdateHostNameBinding(string token, HostNameBindingRequest requestParams)
{
var httpClient = new HttpClient();
string endpoint = $"https://management.azure.com/subscriptions/{requestParams.SubscriptionId}/resourceGroups/{requestParams.ResourceGroupName}/providers/Microsoft.Web/sites/{requestParams.AppName}/slots/{requestParams.Slot}/hostNameBindings/{requestParams.HostName}?api-version=2022-03-01";
var requestBody = new
{
kind = requestParams.Kind,
properties = new
{
azureResourceName = requestParams.AzureResourceName,
azureResourceType = requestParams.AzureResourceType,
customHostNameDnsRecordType = requestParams.CustomHostNameDnsRecordType,
domainId = requestParams.DomainId,
hostNameType = requestParams.HostNameType,
siteName = requestParams.SiteName,
sslState = requestParams.SslState,
thumbprint = requestParams.Thumbprint
}
};
var content = new StringContent(JsonConvert.SerializeObject(requestBody), Encoding.UTF8, "application/json");
httpClient.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Bearer", token);
HttpResponseMessage response = await httpClient.PutAsync(endpoint, content);
if (response.IsSuccessStatusCode)
{
string responseContent = await response.Content.ReadAsStringAsync();
return responseContent;
}
else
{
return $"Error: {response.StatusCode}";
}
}
}
public class HostNameBindingRequest
{
public string Kind { get; set; }
public string AzureResourceName { get; set; }
public string AzureResourceType { get; set; }
public string CustomHostNameDnsRecordType { get; set; }
public string DomainId { get; set; }
public string HostNameType { get; set; }
public string SiteName { get; set; }
public string SslState { get; set; }
public string Thumbprint { get; set; }
public string SubscriptionId { get; set; }
public string ResourceGroupName { get; set; }
public string AppName { get; set; }
public string Slot { get; set; }
public string HostName { get; set; }
}
}