There's always this from Matplotlib's documentation on 'Text rendering with LaTeX':
"Matplotlib can use LaTeX to render text. This is activated by setting text.usetex : True
in your rcParams, or by setting the usetex
property to True on individual Text objects. "
I didn't take that path but you may want to combine it with some of the approaches below.
Getting close without usetex
but using Latex in the plot label
This answer shows you how you can use the dollar sign to place Latex in labels.
The tricks with the ticks are annotated in the code.
This gets you close but you were vague on what you really wanted in a few things and so you'll need to customize some more:
import numpy as np
import matplotlib
import matplotlib.pyplot as plt
import sympy as sp
from scipy.integrate import quad
import math
from matplotlib.patches import Circle
matplotlib.rcParams['font.family'] = ['serif'] # based on https://matplotlib.org/stable/tutorials/text/text_props.html
fig2 = plt.figure(figsize=(10,8))
plt.subplot(1, 1, 1)
plt.plot(2, 5, marker='*', markersize=25, color='gold')
plt.plot(2, -5, marker='*', markersize=25, color='gold')
plt.plot(3, 0, marker='*', markersize=25, color='gold')
#plt.xlabel(r"$x [rg]$", fontsize=20)
#plt.ylabel(r"$y [rg]$", fontsize=20)
circle = Circle((0, 0), 1, color='black')
plt.gca().add_patch(circle)
plt.gca().set_aspect('equal')
plt.axis([-10, 30, -10, 10])
plt.gca().set_ylabel(r'x[rg]' "\n" r'$x[rg]$' "\n" r'$r \slash r_S$',
fontsize=20, rotation = 0, labelpad=35) # rotation based on https://stackabuse.com/rotate-axis-labels-in-matplotlib/
# multiline labels based on https://stackoverflow.com/a/2666270/8508004 (just added for development of example because OP unclear on actual label)
# use of `labelpad` is to complement `set_label_position` use, see next line
plt.gca().yaxis.set_label_position("right") # label on right based on https://stackoverflow.com/a/64363955/8508004
plt.gca().set_xlabel(r"$y [rg]$", fontsize=20, rotation = 0)
plt.gca().minorticks_on() # based on https://stackoverflow.com/a/57280702/8508004
plt.gca().yaxis.set_ticks_position('both')# Ticks on all 4 sides; based on https://stackoverflow.com/a/56577545/8508004
plt.gca().xaxis.set_ticks_position('both') # based on https://stackoverflow.com/a/56577545/8508004
plt.gca().xaxis.set_tick_params(which='both', direction = 'in')# ticks facing inward direction based on https://stackoverflow.com/q/71366709/8508004
plt.gca().yaxis.set_tick_params(which='both', direction = 'in')# ticks facing inward based on https://stackoverflow.com/q/71366709/8508004
plt.tick_params(axis='both', labelsize=20)
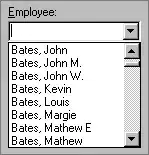