Append Table Data Using CurrentRegion
Before
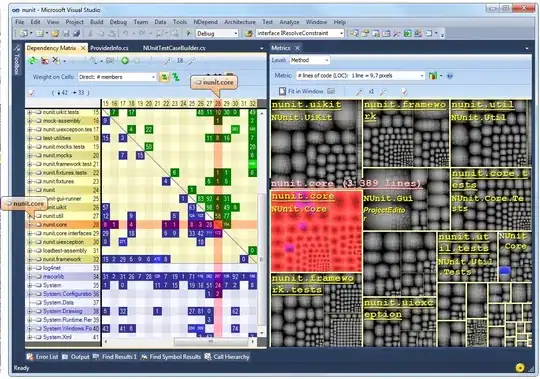
After

The Code
Sub AppendTableData()
Const PROC_TITLE As String = "Append Table Data"
' Define constants.
Const SRC_FIRST_DATA_CELL As String = "A2"
Const DST_SHEET As String = "Monthly"
Const DST_FIRST_DATA_CELL As String = "A2"
' Use an array of names of the sheets that you don't want to be written to.
Dim Exclusions(): Exclusions = Array("Monthly", "Dummy") ' add more
Dim wb As Workbook: Set wb = ThisWorkbook ' workbook containing this code
' Source
Dim sws As Worksheet: Set sws = wb.ActiveSheet
Dim sName As String: sName = sws.Name
If IsNumeric(Application.Match(sName, Exclusions, 0)) Then
MsgBox "The sheet """ & sName & """ is selected." & vbLf _
& "Please select one of the daily sheets.", vbCritical, PROC_TITLE
Exit Sub
End If
Dim sCell As Range: Set sCell = sws.Range(SRC_FIRST_DATA_CELL)
Dim srg As Range
With sCell.CurrentRegion
Set srg = sCell.Resize(.Row + .Rows.Count - sCell.Row, _
.Column + .Columns.Count - sCell.Column)
End With
' Destination
Dim dws As Worksheet: Set dws = wb.Sheets(DST_SHEET)
Dim dCell As Range: Set dCell = dws.Range(DST_FIRST_DATA_CELL)
Dim drrg As Range, IsDataRangeEmpty As Boolean
With dCell.CurrentRegion
Set drrg = dCell.Resize(.Row + .Rows.Count - dCell.Row, _
.Column + .Columns.Count - dCell.Column)
If drrg.Rows.Count = 1 Then ' the destination data range has one row
If Application.CountA(drrg) = 0 Then ' the dest. data range is empty
IsDataRangeEmpty = True
End If
End If
If Not IsDataRangeEmpty Then
Set dCell = drrg.Cells(1).Offset(drrg.Rows.Count)
End If
End With
' Copy.
srg.Copy dCell
' Inform.
MsgBox "The daily data from sheet """ & sName & """ has been copied " _
& "to sheet """ & DST_SHEET & """!", vbInformation, PROC_TITLE
' Debug.Print String(43, "-")
' Debug.Print "Sheet", "Name", "Range Reference"
' Debug.Print String(43, "-")
' Debug.Print "Source", sws.Name, srg.Address
' Debug.Print "Destination", dws.Name, dCell.Address
End Sub
MsgBox
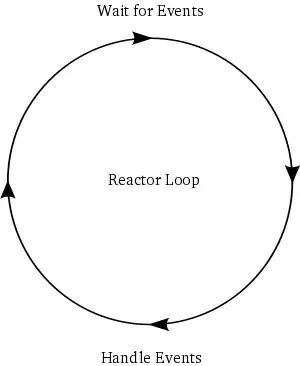
Result in the Immediate Window (Ctrl+G)
-------------------------------------------
Sheet Name Range Reference
-------------------------------------------
Source 2023-08-25 $A$2:$C$3
Destination Monthly $A$2