You are looking for controlling ticks and spines in matplotlib which is being used in your posted code. To start, first try this to have the zero-axes include the ticks and labels (the references are in the comments for the last roughly three lines I added):
import pylab as pl
from numpy import *
pl.style.use('bmh')
# Df = ℝ - {-1, 1}
Df1 = [-4 + k/10 for k in range(28)] # [-4, -1[ par pas de 0.1
Df2 = [-0.8 + k/10 for k in range(18)] # ]-1, 1] par pas de 0.1
Df3 = [1.1 + k/10 for k in range(30)] # ]-1, 4] par pas de 0.1
Df = [Df1, Df2, Df3]
for D in Df:
Y = [(-(x ** 2) + 2 * x) / (x ** 2 - 1) for x in D] # Pour tout x ∈ D, calculer y = f(x)
pl.plot(D, Y, color='red')
pl.legend([ r'$\mathscr{C}_f$'], fontsize = 18) # Cet appel est important : sans, la légende ne s'affichera pas
# Axe des x : de -4 à 4, axe des y : de -6 à 6
pl.axis(xmin=-4, xmax=4, ymin=-6, ymax=6)
pl.axvline(x = 0)
pl.axhline(y = 0)
pl.gca().minorticks_on() # based on https://stackoverflow.com/a/57280702/8508004
pl.gca().spines['bottom'].set_position('zero') #based on https://stackoverflow.com/a/31054289/8508004
pl.gca().spines['left'].set_position('zero'); #based on https://stackoverflow.com/a/31054289/8508004
Here is the result of that code:
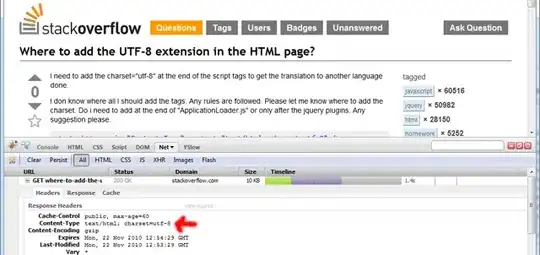
If you'd prefer the zero axes to be more of the solid color added by your axvline()
and axfline()
, you can further adjust that approach to color the axes to match those lines, like so:
import pylab as pl
from numpy import *
pl.style.use('bmh')
# Df = ℝ - {-1, 1}
Df1 = [-4 + k/10 for k in range(28)] # [-4, -1[ par pas de 0.1
Df2 = [-0.8 + k/10 for k in range(18)] # ]-1, 1] par pas de 0.1
Df3 = [1.1 + k/10 for k in range(30)] # ]-1, 4] par pas de 0.1
Df = [Df1, Df2, Df3]
for D in Df:
Y = [(-(x ** 2) + 2 * x) / (x ** 2 - 1) for x in D] # Pour tout x ∈ D, calculer y = f(x)
pl.plot(D, Y, color='red')
pl.legend([ r'$\mathscr{C}_f$'], fontsize = 18) # Cet appel est important : sans, la légende ne s'affichera pas
# Axe des x : de -4 à 4, axe des y : de -6 à 6
pl.axis(xmin=-4, xmax=4, ymin=-6, ymax=6)
pl.axvline(x = 0)
pl.axhline(y = 0)
pl.gca().minorticks_on() # based on https://stackoverflow.com/a/57280702/8508004
pl.gca().spines['bottom'].set_position('zero') #based on https://stackoverflow.com/a/31054289/8508004
pl.gca().spines['left'].set_position('zero') #based on https://stackoverflow.com/a/31054289/8508004
pl.gca().spines['bottom'].set_color('tab:blue') # based on https://stackoverflow.com/a/4762002/8508004
pl.gca().spines['left'].set_color('tab:blue') # based on https://stackoverflow.com/a/4762002/8508004
#pl.gca().xaxis.label.set_color('black') # # not needed(?), also comes from https://stackoverflow.com/a/4762002/8508004
#pl.gca().tick_params(axis='x', colors='tab:blue') # not needed(?), also comes from https://stackoverflow.com/a/4762002/8508004 ;
The result of that code block is an arguably 'more refined' version of the above one:
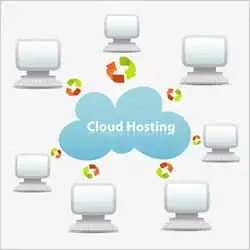
Based on the original post title and text, I thought you meant have the ticks not just be on the left and bottom. For others ending up here with that issue, try this below to get the ticks at all the axes of the plot (the resources it is based on are in the comments of the five lines added):
import pylab as pl
from numpy import *
pl.style.use('bmh')
# Df = ℝ - {-1, 1}
Df1 = [-4 + k/10 for k in range(28)] # [-4, -1[ par pas de 0.1
Df2 = [-0.8 + k/10 for k in range(18)] # ]-1, 1] par pas de 0.1
Df3 = [1.1 + k/10 for k in range(30)] # ]-1, 4] par pas de 0.1
Df = [Df1, Df2, Df3]
for D in Df:
Y = [(-(x ** 2) + 2 * x) / (x ** 2 - 1) for x in D] # Pour tout x ∈ D, calculer y = f(x)
pl.plot(D, Y, color='red')
pl.legend([ r'$\mathscr{C}_f$'], fontsize = 18) # Cet appel est important : sans, la légende ne s'affichera pas
# Axe des x : de -4 à 4, axe des y : de -6 à 6
pl.axis(xmin=-4, xmax=4, ymin=-6, ymax=6)
pl.axvline(x = 0)
pl.axhline(y = 0)
pl.gca().minorticks_on() # based on https://stackoverflow.com/a/57280702/8508004
pl.gca().yaxis.set_ticks_position('both')# Ticks on all 4 sides; based on https://stackoverflow.com/a/56577545/8508004
pl.gca().xaxis.set_ticks_position('both') # based on https://stackoverflow.com/a/56577545/8508004
pl.gca().xaxis.set_tick_params(which='both', direction = 'in')# ticks facing inward direction based on https://stackoverflow.com/q/71366709/8508004
pl.gca().yaxis.set_tick_params(which='both', direction = 'in')# ticks facing inward based on https://stackoverflow.com/q/71366709/8508004;
The result of the bottom code block:
