The easiest way is probably to use Pandoc. Simply type following command to your Command Prompt:
pandoc super-secret-notebook.ipynb -s -o export.docx
As @I_K suggested, you can export it to a PDF file and then save it as a Word document. Since you have the tag python in your question, I assume that you are looking for a Python solution.
Here is an example Python script doing this (requires nbconvert
, pywin32
, Pandoc and Microsoft Word installed):
import os
from pathlib import Path
import subprocess
import win32com.client
PATH_TO_NBCONVERT_EXE = r"C:\apps\python-3.11.1\Scripts\jupyter-nbconvert.exe"
cwd = Path(__file__).parent.resolve()
SCRIPT_PATH = os.path.join(cwd, "super-secret-notebook.ipynb")
FILE_PATH = os.path.join(cwd, "export.docx")
def main():
subprocess.run(
["jupyter-nbconvert", "--execute", "--to", "pdf", SCRIPT_PATH],
cwd=cwd,
shell=True
)
word = win32com.client.Dispatch("Word.Application")
doc = word.Documents.Add(SCRIPT_PATH.replace(".ipynb", ".pdf"))
doc.SaveAs(FILE_PATH, FileFormat=12)
doc.Close()
word.Quit()
if __name__ == "__main__":
main()
The output looks a bit different than simply using Pandoc:
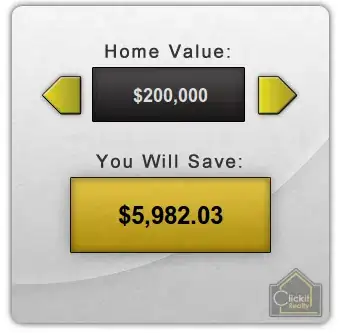
Please note that the script above (and below) is executing nbconvert
in the command prompt. You could also use the package nbconvert
in Python itself.
Why do you want a Word document? PDF files are easier to distribute. Anyways, if you just need a PDF file, you can generate the file in your Command Prompt as well (without a script):
jupyter-nbconvert --execute --to pdf super-secret-notebook.ipynb
It still requires nbconvert
and Pandoc installed.
You can also export to an HTML file and then save it as a Word document. (not requiring Pandoc)
Following Python script executes and exports a Notebook file to HTML and then saves it as a Word document. Formatting is not very useful though.
import os
from pathlib import Path
import subprocess
import win32com.client
PATH_TO_NBCONVERT_EXE = r"C:\apps\python-3.11.1\Scripts\jupyter-nbconvert.exe"
cwd = Path(__file__).parent.resolve()
SCRIPT_PATH = os.path.join(cwd, "super-secret-notebook.ipynb")
FILE_PATH = os.path.join(cwd, "export.docx")
def main():
subprocess.run(
["jupyter-nbconvert", "--execute", "--to", "html", SCRIPT_PATH],
cwd=cwd,
shell=True
)
word = win32com.client.Dispatch("Word.Application")
doc = word.Documents.Add(SCRIPT_PATH.replace(".ipynb", ".html"))
doc.SaveAs(FILE_PATH, FileFormat=12)
doc.Close()
word.Quit()
if __name__ == "__main__":
main()
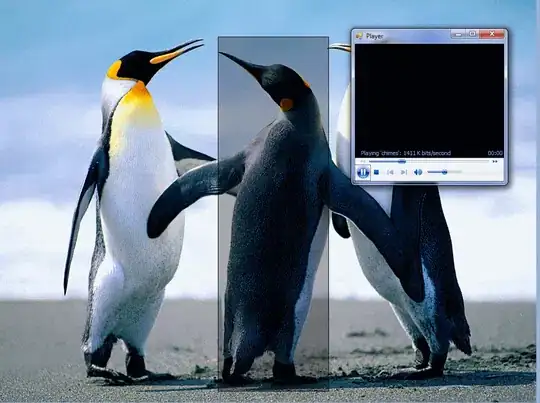
Off-topic: Just some note if anyone comes across this answer and is wondering where to find the constants for the FileFormat
parameter: