Since the timing seems to be rather important. I've moved the comparisons between the various methods to this, Community Wiki, answer.
The data used are simply lists of consecutive integers, with a single non-consecutive point, and they're generated via
d : dat[n_Integer?Positive] := (d = {1}~Join~Range[1, n])
d : dat[n_Integer?Positive, p_Integer?Positive] /; p <= n :=
Range[1, p]~Join~{p}~Join~Range[p + 1, n]
where the first form of dat[n]
is equivalent to dat[n, 1]
. The timing code is simple:
Clear[consQTiming]
Options[consQTiming] = {
NonConsecutivePoints -> {10, 25, 50, 100, 250,500, 1000}};
consQTiming[fcns__, OptionPattern[]]:=
With[{rnd = RandomInteger[{1, #}, 100]},
With[{fcn = #},
Timing[ fcn[dat[10000, #]] & /@ rnd ][[1]]/100
] & /@ {fcns}
] & /@ OptionValue[NonConsecutivePoints]
It generates 100 random integers between 1 and each of {10, 25, 50, 100, 250, 500, 1000}
and dat
then uses each of those random numbers as the non-consecutive point in a list 10,000 elements long. Each consQ
implementation is then applied to each list produced by dat
, and the results are averaged. The plotting function is simply
Clear[PlotConsQTimings]
Options[PlotConsQTimings] = {
NonConsecutivePoints -> {10, 25, 50, 100, 250, 500, 1000}};
PlotConsQTimings[timings : { _?VectorQ ..}, OptionPattern[]] :=
ListLogLogPlot[
Thread[{OptionValue[NonConsecutivePoints], #}] & /@ Transpose[timings],
Frame -> True, Joined -> True, PlotMarkers -> Automatic
]
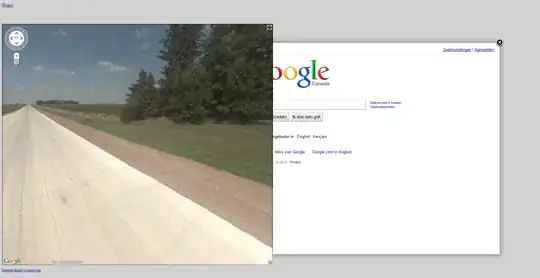
I timed the following functions consQSzabolcs1
, consQSzabolcs2
, consQBrett
, consQRCollyer
, consQBelisarius
, consQWRFold
, consQWRFold2
, consQWRFold3
, consQWRMatch
, and MrWizard's version of consQBelisarius
.
In ascending order of the left most timing: consQBelisarius
, consQWizard
, consQRCollyer
, consQBrett
, consQSzabolcs1
, consQWRMatch
, consQSzabolcs2
, consQWRFold2
, consQWRFold3
,and consQWRFold
.
Edit: Reran all of the functions with timeAvg
(the second one) instead of Timing
in consQTiming
. I did still average over 100 runs, though. For the most part, there was any change, except for the lowest two where there is some variation from run to run. So, take those two lines with a grain of salt as they're timings are practically identical.
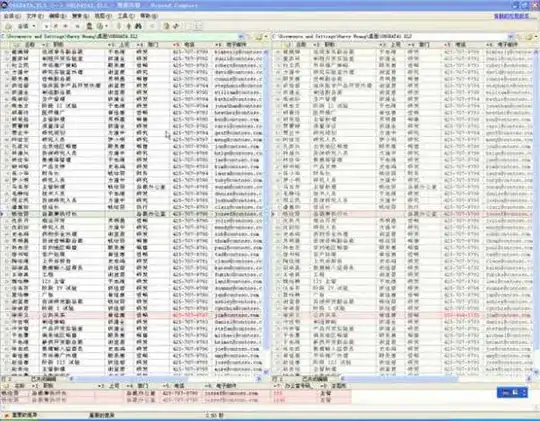