In JAVA for example these tools provide many algorithms for image segmentation:
ImageJ
http://rsbweb.nih.gov/ij/
Fiji
http://fiji.sc/wiki/index.php/Fiji
Rapidminer IMMI
http://www.burgsys.com/image-mining
Marvin Framework
http://marvinproject.sourceforge.net/
COMPLEMENT
Even being generic, I think it is possible to answer this question in some sense. Since this question is closed, I gonna complement @radim-burget answer for those people that get here looking for a simple example of image segmentation in Java.
Image Segmentation is an image processing task and is handled by most image processing frameworks. In the example below I'm using Marvin Framework.
Algorithm to segment diagram elements:
- Load image and binarize
- Apply morphological erosion to remove lines, texts, etc
- Apply floodfill segmentation to get segments
- Draw the segments in the original image.
Input:
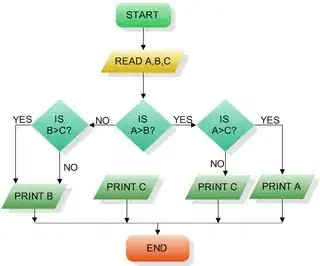
After Erosion:
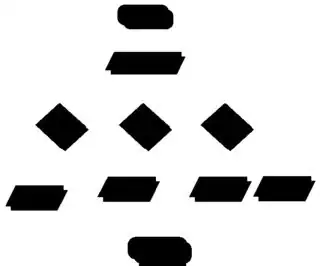
Result:
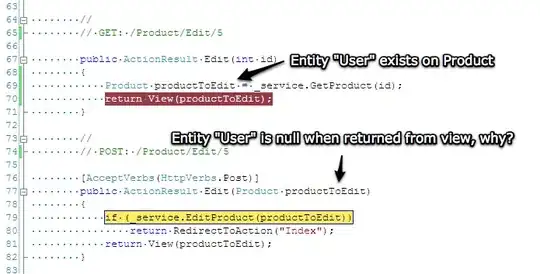
Source Code:
import static marvin.MarvinPluginCollection.*;
public class SegmentDiagram {
public SegmentDiagram(){
MarvinImage originalImage = MarvinImageIO.loadImage("./res/diagram.png");
MarvinImage image = originalImage.clone();
MarvinImage binImage = MarvinColorModelConverter.rgbToBinary(image, 250);
morphologicalErosion(binImage.clone(), binImage, MarvinMath.getTrueMatrix(5, 5));
image = MarvinColorModelConverter.binaryToRgb(binImage);
MarvinSegment[] segments = floodfillSegmentation(image);
for(int i=1; i<segments.length; i++){
MarvinSegment seg = segments[i];
originalImage.drawRect(seg.x1, seg.y1, seg.width, seg.height, Color.red);
originalImage.drawRect(seg.x1+1, seg.y1+1, seg.width, seg.height, Color.red);
}
MarvinImageIO.saveImage(originalImage, "./res/diagram_segmented.png");
}
public static void main(String[] args) {
new SegmentDiagram();
}
}
The shape recognition is another topic, already discussed on Stack Overflow:
2D Shape recognition algorithm - looking for guidance