that example does not work for you because author have not shown piece of code responsible for setting vertexes.
Here is my example(it is long, but that's opengl...)
NOTE - remember to setUp viewport correctly.
public static void drawGradientRectangle(GL10 gl, float centerX, float centerY,
float width, float height) {
gl.glPushMatrix();
gl.glDisable(GL10.GL_TEXTURE_2D);
gl.glEnableClientState(GL10.GL_COLOR_ARRAY);
gl.glEnableClientState(GL10.GL_VERTEX_ARRAY); //just in case if you have not done that before
gl.glFrontFace(GL10.GL_CCW); //Set the face
gl.glTranslatef(centerX, centerY, 0);
if (width != 1 || height != 1) {
gl.glScalef(width, height, 1);
}
gl.glVertexPointer(2, GL10.GL_FLOAT, 0, GLDrawConstants.vertexBuffer0_5);
gl.glColorPointer(4, GL10.GL_FLOAT, 0, GLDrawConstants.gradOrangeWhiteBuffer);
// Draw the vertices as triangle strip
gl.glDrawArrays(GL10.GL_TRIANGLE_STRIP, 0, 4);
gl.glDisableClientState(GL10.GL_COLOR_ARRAY);
gl.glEnable(GL10.GL_TEXTURE_2D);
gl.glPopMatrix();
}
GLDrawConstants class:
public class GLDrawConstants {
public static final FloatBuffer gradOrangeWhiteBuffer;
public static final FloatBuffer vertexBuffer0_5;
private static final float vertices0_5[] = {
-0.5f, -0.5f,// Bottom Left
0.5f, -0.5f,// Bottom right
-0.5f, 0.5f,// Top Left
0.5f, 0.5f// Top Right
};
private static final float gradOrangeWhiteColor[] = {
255/255f, 239/255f, 196/255f, 0f, // Bottom Left
255/255f, 239/255f, 196/255f, 0f, // Bottom right
250/255f, 200/255f, 62/255f, 0.3f, // Top Left
250/255f, 200/255f, 62/255f, 0.3f // Top Right
};
static {
gradOrangeWhiteBuffer = WDUtils.floatBuffer(gradOrangeWhiteColor);
vertexBuffer0_5 = WDUtils.floatBuffer(vertices0_5);
}
}
WDUtils class:
public class WDUtils {
/**
* Make a direct NIO FloatBuffer from an array of floats
*
* @param arr
* The array
* @return The newly created FloatBuffer
*/
public static final FloatBuffer floatBuffer(float[] arr) {
ByteBuffer bb = ByteBuffer.allocateDirect(arr.length * 4);
bb.order(ByteOrder.nativeOrder());
FloatBuffer fb = bb.asFloatBuffer();
fb.put(arr);
fb.position(0);
return fb;
}
}
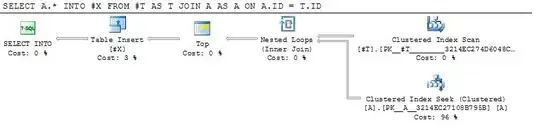