Check the TableLayout. To split your text you could split your text after 1/3 of your count of chars. Of cource you have to split the text at one whitespace charecter. UPDATE: See also my example code at the end of my posting.
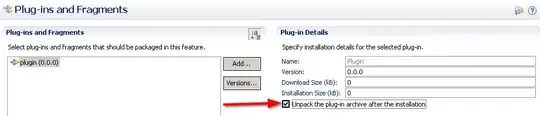
<?xml version="1.0" encoding="utf-8"?>
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:stretchColumns="1">
<TableRow>
<TextView
android:text="@string/table_layout_4_open"
android:padding="3dip" />
<TextView
android:text="@string/table_layout_4_open_shortcut"
android:gravity="right"
android:padding="3dip" />
</TableRow>
<TableRow>
<TextView
android:text="@string/table_layout_4_save"
android:padding="3dip" />
<TextView
android:text="@string/table_layout_4_save_shortcut"
android:gravity="right"
android:padding="3dip" />
</TableRow>
</TableLayout>
For splitting you can test this code. The algorithem could maybe a little better be nearer to the split boundaries, but it works.
public static String[] getRows(String text, int rows) {
// some checks
if(text==null)
throw new NullPointerException("text was null!");
if(rows<0 && rows > 10)
throw new IllegalArgumentException("rows must be between 1 and 10!");
if(rows==1)
return new String[] { text };
// some init stuff
int len=text.length();
int splitOffset=0;
String[] ret=new String[rows];
Pattern whitespace = Pattern.compile("\\w+");
// do the work
for(int row=1;row<rows;row++) {
int end;
int searchOffset=len/rows*row;
// search next white space
Matcher matcher = whitespace.matcher(text.substring(searchOffset));
if(matcher.find() && !matcher.hitEnd()) {
// splitting on white space
end=matcher.end()+searchOffset;
} else {
// hard splitting if there are no white spaces
end=searchOffset;
}
ret[row-1]=text.substring(splitOffset, end);
splitOffset=end;
}
// put the remaing into the last element
ret[rows-1]=text.substring(splitOffset);
return ret;
}