Assume a table called Colors with fields ID
, Color
, and ColorIndex
of types int
, varchar
, and int
respectively. I also assume the OP means prev / after based on an ordering of the ID
field in asc
order.
You could do this without a cursor, and use a while loop...but it definately isn't set based:
DECLARE @MyID int
DECLARE @CurrentIndex int
DECLARE @CurrentColor varchar(50)
DECLARE @PreviousColor varchar(50)
SET @CurrentIndex = (SELECT 0)
SET @MyID = (SELECT TOP 1 ID FROM Colors ORDER BY ID ASC)
SET @CurrentColor = (SELECT '')
SET @PreviousColor = (SELECT Color FROM Colors WHERE ID = @MyID)
WHILE (@MyID IS NOT NULL)
BEGIN
IF (@CurrentColor <> @PreviousColor)
BEGIN
SET @PreviousColor = (SELECT Color FROM Colors WHERE ID = @MyID)
SET @CurrentIndex = (SELECT @CurrentIndex + 1)
UPDATE Colors SET ColorIndex = @CurrentIndex WHERE ID = @MyID
END
ELSE
BEGIN
UPDATE Colors SET ColorIndex = @CurrentIndex WHERE ID = @MyID
SET @PreviousColor = (SELECT Color FROM Colors WHERE ID = @MyID)
END
SET @MyID = (SELECT TOP 1 ID FROM Colors WHERE ID > @MyID ORDER BY ID ASC)
SET @CurrentColor = (SELECT Color FROM Colors WHERE ID = @MyID)
END
The result after execution:
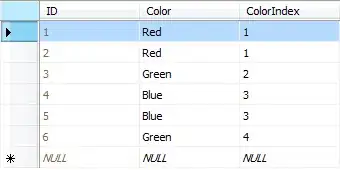
Performance wasn't too shabby as long as ID and color are indexed. The plus side is it is a bit faster then using a regular old CURSOR and it's not as evil. Solution supports SQL 2000, 2005, and 2008 (being that you are using SQL 2000 which did not support CTEs).