Yes you can have regular classes and no they are not all related to a UI element. This works pretty much like normal Java. So in Eclipse you can create a new class like in the image and follow the one page wizard.
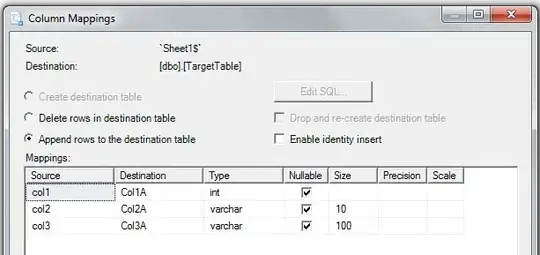
You will end up with some code like the below (I added a few bits for the example):
package this.is.your.package;
public class Person{
private int age;
public void setAge(int _age)
{
age = _age;
}
}
You can then build methods and other stuff as normal. As for instantiating or accessing your class you will probably have to make it public for the activities to get it. However there are a many number of different ways of doing this but for the example above I could do.
public class MyActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
Person me = new Person();
me.setAge(22); //feels old
}
As you can see this is all pretty normal.