I know this is an old post, but I ran across this today.
https://learn.microsoft.com/en-us/dotnet/api/system.reflection.assemblyinformationalversionattribute?view=net-5.0
Apparently this attribute has been present since .Net 1.1. You can also use non-standard version in FileVersion, but you will get a compiler warning. AssemblyVersion won't compile if it isn't in the correct format. InformationalVersion can be apparently anything.
If you set a non-standard FileVersion, Windows Properties tries to parse it as a normal version, so in my example screenshots below, the '-RC1' gets dropped. If you put in something nonsensical (like 'blarg'), then it just becomes '0.0.0.0' for the file version.
In your code, if you are a WinForms project, you can access the new information version from Application.ProductVersion
. For non-WinForms, to avoid loading the WinForms DLL into memory, you can basically copy the code from the reference source code for Application.ProductVersion. Either way, it is a string, so if you want to do any kind of comparison logic in your code, if you can't immediately parse it as Version object, you will need to write your own parser / comparer.
Assembly entryAssembly = Assembly.GetEntryAssembly();
if (entryAssembly != null) {
object[] attrs = entryAssembly.GetCustomAttributes(typeof(AssemblyInformationalVersionAttribute), false);
if (attrs != null && attrs.Length > 0) {
productVersion = ((AssemblyInformationalVersionAttribute)attrs[0]).InformationalVersion;
}
}

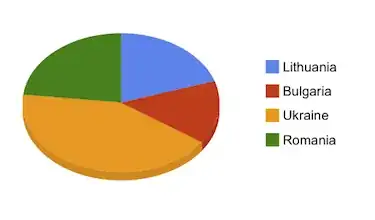