Slightly different problem, that is if you want two 1D array with shape (n,)
into (2,n)
, then you have the following options:
import numpy as np
np.r_[[a], [a]]
np.stack([a, a])
np.vstack([a, a])
np.concatenate([[a], [a]])
np.array([a, a])
The fastest way is to use plain numpy.array
:
import numpy
import perfplot
perfplot.show(
setup=lambda n: numpy.random.rand(n),
kernels=[
lambda a: numpy.r_[[a], [a]],
lambda a: numpy.stack([a, a]),
lambda a: numpy.vstack([a, a]),
lambda a: numpy.concatenate([[a], [a]]),
lambda a: numpy.array([a, a]),
],
labels=["r_", "stack", "vstack", "concatenate", "array"],
n_range=[2 ** k for k in range(19)],
xlabel="len(a)",
)
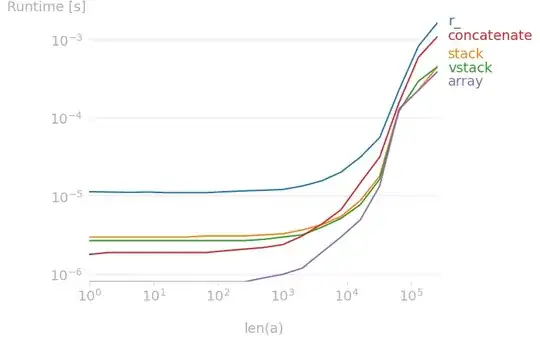