tl;dr
LocalDate previousMonday =
LocalDate.now( ZoneId.of( "America/Montreal" ) )
.with( TemporalAdjusters.previous( DayOfWeek.MONDAY ) );
java.time
Both the java.util.Calendar class and the Joda-Time library have been supplanted by the java.time framework built into Java 8 and later. See Oracle Tutorial. Much of the java.time functionality has been back-ported to Java 6 & 7 in ThreeTen-Backport and further adapted to Android in ThreeTenABP. With modern Android tooling and its "API desugaring", you need not add any library.
The java.time.LocalDate
class represents a date-only value without time-of-day and without time zone.
Determining today's date requires a time zone, a ZoneId
.
ZoneId zoneId = ZoneId.of( "America/Montreal" );
LocalDate today = LocalDate.now( zoneId );
The TemporalAdjuster
interface (see Tutorial) is a powerful but simple way to manipulate date-time values. The TemporalAdjusters
class (note the plural) implements some very useful adjustments. Here we use previous( DayOfWeek)
.
The handy DayOfWeek
enum makes it easy to specify a day-of-week.
LocalDate previousMonday = today.with( TemporalAdjusters.previous( DayOfWeek.MONDAY ) );
If today is Monday, and you want to use today rather than a week ago, call previousOrSame
.
About java.time
The java.time framework is built into Java 8 and later. These classes supplant the troublesome old legacy date-time classes such as java.util.Date
, Calendar
, & SimpleDateFormat
.
To learn more, see the Oracle Tutorial. And search Stack Overflow for many examples and explanations. Specification is JSR 310.
The Joda-Time project, now in maintenance mode, advises migration to the java.time classes.
You may exchange java.time objects directly with your database. Use a JDBC driver compliant with JDBC 4.2 or later. No need for strings, no need for java.sql.*
classes. Hibernate 5 & JPA 2.2 support java.time.
Where to obtain the java.time classes?
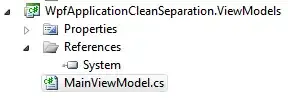
The ThreeTen-Extra project extends java.time with additional classes. This project is a proving ground for possible future additions to java.time. You may find some useful classes here such as Interval
, YearWeek
, YearQuarter
, and more.