availability of parent allows to iterate, without a stack: this algorithm assume each node has Parent, FirstChild, NextSibling
#include <stdio.h>
struct Node {
int Data; Node *FirstChild, *NextSibling, *Parent;
};
void use_data(Node *node) {
printf("%d\n", node->Data);
}
int main_visit_post(int, char **) {
Node G[] = {
{0, G+1, 0, 0},
{1, G+2, G+3, G+0},
{2, 0, G+4, G+1},
{3, G+8, 0, G+0},
{4, G+5, G+7, G+1},
{5, 0, G+6, G+4},
{6, 0, 0, G+4},
{7, 0, 0, G+1},
{8, G+9, 0, G+3},
{9, 0, 0, G+8},
};
Node *node = G, *next;
while (node) {
next = node->FirstChild;
if (!next) {
use_data(node);
next = node->NextSibling;
if (!next) {
while (node->Parent) {
next = node->Parent;
use_data(next);
if (next->NextSibling) {
next = next->NextSibling;
break;
}
else {
node = next;
next = 0;
}
}
}
}
node = next;
}
return 0;
}
this tree get this sequence: 2,5,6,4,7,1,9,8,3,0
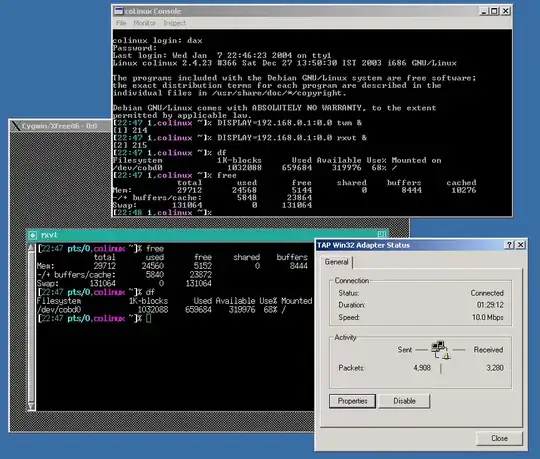