Try out my library TableIt
NOTE: This answer is already posted on this question.
I just made a library for this that I think could really help. It is extremely simple, that's why I think you should use it. It is called TableIT.
Basic Use
To use it, first follow the download instructions on the GitHub Page.
Then import it:
import TableIt
Then make a list of lists where each inner list is a row:
table = [
[4, 3, "Hi"],
[2, 1, 808890312093],
[5, "Hi", "Bye"]
]
Then all you have to do is print it:
TableIt.printTable(table)
This is the output you get:
+--------------------------------------------+
| 4 | 3 | Hi |
| 2 | 1 | 808890312093 |
| 5 | Hi | Bye |
+--------------------------------------------+
Field Names
You can use field names if you want to (if you aren't using field names you don't have to say useFieldNames=False because it is set to that by default):
TableIt.printTable(table, useFieldNames=True)
From that you will get:
+--------------------------------------------+
| 4 | 3 | Hi |
+--------------+--------------+--------------+
| 2 | 1 | 808890312093 |
| 5 | Hi | Bye |
+--------------------------------------------+
There are other uses to, for example you could do this:
import TableIt
myList = [
["Name", "Email"],
["Richard", "richard@fakeemail.com"],
["Tasha", "tash@fakeemail.com"]
]
TableIt.print(myList, useFieldNames=True)
From that:
+-----------------------------------------------+
| Name | Email |
+-----------------------+-----------------------+
| Richard | richard@fakeemail.com |
| Tasha | tash@fakeemail.com |
+-----------------------------------------------+
Or you could do:
import TableIt
myList = [
["", "a", "b"],
["x", "a + x", "a + b"],
["z", "a + z", "z + b"]
]
TableIt.printTable(myList, useFieldNames=True)
And from that you get:
+-----------------------+
| | a | b |
+-------+-------+-------+
| x | a + x | a + b |
| z | a + z | z + b |
+-----------------------+
Colors
You can also use colors.
You use colors by using the color option (by default it is set to None) and specifying RGB values.
Using the example from above:
import TableIt
myList = [
["", "a", "b"],
["x", "a + x", "a + b"],
["z", "a + z", "z + b"]
]
TableIt.printTable(myList, useFieldNames=True, color=(26, 156, 171))
Then you will get:
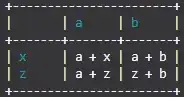
Please note that printing colors might not work for you but it does works the exact same as the other libraries that print colored text. I have tested and every single color works. The blue is not messed up either as it would if using the default 34m
ANSI escape sequence (if you don't know what that is it doesn't matter). Anyway, it all comes from the fact that every color is RGB value rather than a system default.
More Info
For more info check the GitHub Page