- not your are simply wrong, nor there reinvent the wheels by re_implementing one Comparator integrated in the API with another Comparator, another ways is implementing RowFilter, example for RowFilter

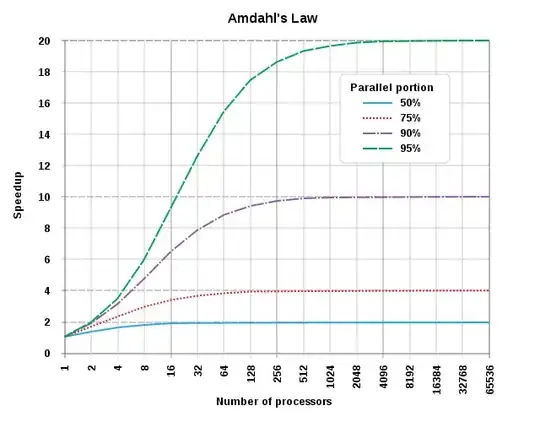

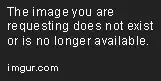
from code
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
import javax.swing.table.*;
public class TableBoolean extends JFrame {
private final static String LETTERS = "ABCDEFGHIJKLMNOPQRSTUVWXYZ";
private static final long serialVersionUID = 1L;
private JTable table;
private DefaultTableModel model;
public TableBoolean() {
Object[][] data = {{"A", new Boolean(false), 2, 1}, {"B", new Boolean(true), 1, 3},
{"A", new Boolean(false), 1, 5}, {"B", new Boolean(true), 3, 1},
{"A", new Boolean(false), 2, 3}, {"B", new Boolean(true), 2, 4},
{"A", new Boolean(false), 5, 2}, {"B", new Boolean(true), 7, 1}};
String[] columnNames = {"String", "Boolean", "Integer", "Integer"};
model = new DefaultTableModel(data, columnNames) {
private static final long serialVersionUID = 1L;
@Override
public Class getColumnClass(int column) {
return getValueAt(0, column).getClass();
}
@Override
public boolean isCellEditable(int row, int column) {
return true;
}
};
table = new JTable(model);
TableRowSorter<TableModel> sorter = new TableRowSorter<TableModel>(table.getModel());
table.setRowSorter(sorter);
table.setPreferredScrollableViewportSize(table.getPreferredSize());
JScrollPane scrollPane = new JScrollPane(table);
add(scrollPane);
/*JButton button = new JButton("Add Row");
button.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
Object[] newRow = new Object[2];
int row = table.getRowCount() + 1;
newRow[0] = LETTERS.substring(row - 1, row);
newRow[1] = (row % 2 == 0) ? new Boolean(true) : new Boolean(false);
model.addRow(newRow);
}
});
add(button, BorderLayout.SOUTH);*/
}
public static void main(String[] args) {
SwingUtilities.invokeLater(new Runnable() {
@Override
public void run() {
TableBoolean frame = new TableBoolean();
frame.setDefaultCloseOperation(EXIT_ON_CLOSE);
frame.pack();
frame.setVisible(true);
}
});
}
}