if you want to render PDF content and ignoring the orginal format (boldness, font size.. etc) you can parse PDF using any PDF parser(PDFBox, Tika .. etc) and then set the string result to any text Component (JTextFiled or JTextArea).
otherwise you should use PDF rendering library. there are some commercial libraries for that.
but there is small trick i was used in my last project to display PDF in my own panel, like this:
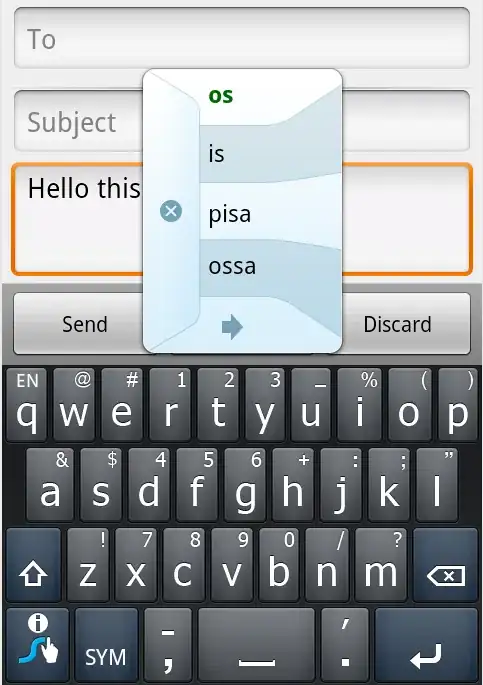
the idea is use embedded web component in your application , and then pass the file path to this component, then web rendering component will load the appropriate PDF rendering tool available in your machine, in my case the machine have acrobat reader.
i use this library Native Swing from DJ project:
http://djproject.sourceforge.net/ns/
just make web browser:
private JWebBrowser fileBrowser = new JWebBrowser();
and control the browser appearance, then add the browser to your main panel ( its layout is BorderLayout)
fileBrowser.setBarsVisible(false);
fileBrowser.setStatusBarVisible(false);
fileRenderPanel.add(fileBrowser, BorderLayout.CENTER);
then if you to render PDF use:
fileBrowser.navigate(filePath);
if you want to highlight some keyword in the PDF:
fileBrowser.navigate(filePath + "#search= " + keyword + ""); // work on acrobat reader only
if you want to render other text (plain, html):
fileBrowser.setHTMLContent(htmlContent);