So a simple solution here would be to break it up into parts.
Each time you make a statement it can return the newly created item.
Lets look at your example -
<li data-target="c1.html"><span>I. </span><span>Het tijdperk der goden</span></li>
We'll take a base object to start with - let us say for example the <ul>
element that you are wanting to append to -
This assumes a <ul>
element like <ul id="someList"></ul>
to be in the HTML markup.
var myList = $("#someList");
And append to it another new <li>
element returning it to a new object
var newElement = myList.append('<li data-target="c1.html"></li>');
Great! we got this far - now we add the <span>
's
var firstSpan = newElement.append('<span></span>').text('I. ');
var secondSpan = newElement.append('<span></span>').text('Het tijdperk der goden');
I've gone about this in a very spread out way - there is no need to perform each operation in a different command. jQuery has a fantastic feature called chaining
.
What chaining means (as the name imply's) is that you can chain functions together. I already gave an example in the code above
var firstSpan = newElement.append('<span></span>').text('I. ')
As you can see I am appending the <span>
element and immediately after calling the text()
function. This is possible because most if not all built-in jQuery functions (an indeed any well built plugins) will return the object itself when it exits.
For example -
var coldBeer = new Object();
coldBeer.drink = function(){
// gulp...gulp...
console.log('gulp...gulp...')
return this;
}
coldBeer.refill = function(){
// drunk...drunk...
console.log('drunk...drunk...')
return this;
}
Would allow us to do this -
coldBeer.drink().refill().drink().refill().drink();
because each function (* hiccup *) would return another cold beer!
Cheers!
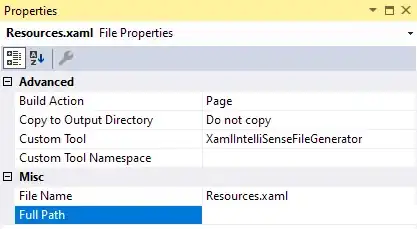
(source: howthewestisfound.com)