As said before, you should use the fill_between
function from pyplot.
And to fill the desired area under the curve, I recommend using the where
argument that provide a filter that fit your data:
import numpy as np
from matplotlib import pyplot as plt
def f(t):
return t * t
t = np.arange(-4,4,1/40)
#Print the curve
plt.plot(t,f(t))
#Fill under the curve
plt.fill_between(
x= t,
y1= f(t),
where= (-1 < t)&(t < 1),
color= "b",
alpha= 0.2)
plt.show()
The where
argument accepts an array of boolean. So you can use numpy arrays operations on boolean to make it easy. As you can see in the example, I simply used : (-1 < t)&(t < 1)
. More details on boolean array here : http://www.math.buffalo.edu/~badzioch/MTH337/PT/PT-boolean_numpy_arrays/PT-boolean_numpy_arrays.html
You can play around with the parameters alpha
(opacity) and color
to make it look better.
Here is the desired result :
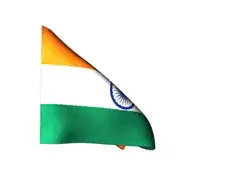
The documentation of fill_between
is available here :
https://matplotlib.org/3.5.1/api/_as_gen/matplotlib.pyplot.fill_between.html