It appears that nobody has mentioned the built-in pyplot method for cycling properties yet. So here it is:
import numpy as np
import matplotlib.pyplot as plt
from cycler import cycler
x = np.linspace(0,3,20)
y = np.sin(x)
fig = plt.figure()
plt.gca().set_prop_cycle(marker=['o', '+', 'x', '*', '.', 'X']) # gca()=current axis
for q,p in zip(x,y):
plt.plot(q,p, linestyle = '')
plt.show()
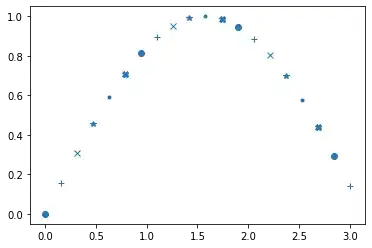
However, this way you lose the color cycle. You can add back color by multiplying or adding a color cycler
and a marker cycler
object, like this:
fig = plt.figure()
markercycle = cycler(marker=['o', '+', 'x', '*', '.', 'X'])
colorcycle = cycler(color=['blue', 'orange', 'green', 'magenta'])
# Or use the default color cycle:
# colorcycle = cycler(color=plt.rcParams['axes.prop_cycle'].by_key()['color'])
plt.gca().set_prop_cycle(colorcycle * markercycle) # gca()=current axis
for q,p in zip(x,y):
plt.plot(q,p, linestyle = '')
plt.show()
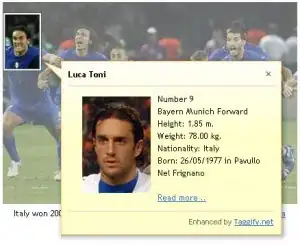
When adding cycles, they need to have the same length, so we only use the first four elements of markercycle
in that case:
plt.gca().set_prop_cycle(colorcycle + markercycle[:4]) # gca()=current axis
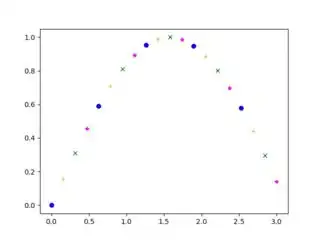