As Marek points out in his answer you have the css attribute id wrong, you need to use -fx-background-position: right center;
Here is a short example which demonstrates adding a picture to the right side of a TextField using CSS:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.TextField;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class TextFieldCssSample extends Application {
@Override public void start(Stage stage) throws Exception {
TextField textField = new TextField();
textField.setId("textField");
StackPane layout = new StackPane();
layout.getChildren().addAll(textField);
layout.getStylesheets().add(this.getClass().getResource("textfield.css").toExternalForm());
stage.setScene(new Scene(layout));
stage.show();
}
public static void main(String[] args) { launch(args); }
}
Css File:
/* textfield.css
place in same location as TextFieldCssSample.java and ensure build system copies it to output directory
image used courtesy of creative commons attribution license: http://www.rockettheme.com/blog/design/1658-free-halloween-icon-pack1
*/
.root {
-fx-padding: 15;
-fx-background-color: cornsilk;
}
#textField {
-fx-background-image:url('http://icons.iconarchive.com/icons/rockettheme/halloween/32/pumpkin-icon.png');
-fx-background-repeat: no-repeat;
-fx-background-position: right center;
-fx-font-size: 20;
}
Sample output:
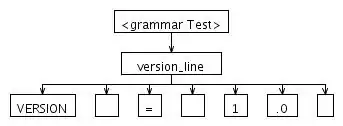
If my png image is a local file in my jar file, how will I access or refer to it?
According to the uri section of the css reference:
"address can be an absolute URI . . . or relative to the location of the CSS file."
For example
- a) Put the css and image files in the same location in the jar file and reference with just
url('pumpkin-icon.png');
OR
- b) Put the image files in an
images
directory underneath the directory holding the css, and reference like url('images/pumpkin-icon.png');
OR
- c) Put the image files in an
images
directory at the root of your jar, and reference like url('/images/pumpkin-icon.png');
Do not use a relative reference which uses a ..
parent specifier e.g. ../images/pumpkin-icon.png
as, although it works for a disk file, the ..
specifier is not a valid jar protocol path and will not extract a file from a jar.