There are probably other ways to achieve this same result, like converting the text to a shape and using a AffineTransformation
, but this is the one that came to hand...
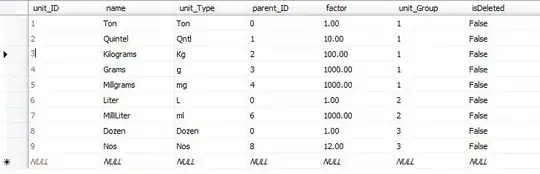
public class StretchText {
public static void main(String[] args) {
new StretchText();
}
public StretchText() {
EventQueue.invokeLater(new Runnable() {
@Override
public void run() {
try {
UIManager.setLookAndFeel(UIManager.getSystemLookAndFeelClassName());
} catch (ClassNotFoundException | InstantiationException | IllegalAccessException | UnsupportedLookAndFeelException ex) {
}
JFrame frame = new JFrame("Test");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setLayout(new BorderLayout());
frame.add(new TestPane());
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
});
}
public class TestPane extends JPanel {
private BufferedImage imgTet;
public TestPane() {
Font font = UIManager.getFont("Label.font");
FontMetrics fm = getFontMetrics(font);
String text = "This is a test";
int width = fm.stringWidth(text);
int height = fm.getHeight();
imgTet = new BufferedImage(width, height, BufferedImage.TYPE_INT_ARGB);
Graphics2D g2d = imgTet.createGraphics();
g2d.setColor(Color.BLACK);
g2d.drawString(text, 0, fm.getAscent());
g2d.dispose();
}
@Override
public Dimension getPreferredSize() {
return new Dimension(200, 200);
}
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
Graphics2D g2d = (Graphics2D) g;
g2d.drawImage(imgTet, 0, 0, getWidth(), imgTet.getHeight(), this);
g2d.dispose();
}
}
}