I can't quite tell what you want from your question.
Do you want all of the plots to have the same data limits?
If so, use shared axes (I'm using subplots
here, but you can avoid it if you want to stick to matlab-style code):
import matplotlib.pyplot as plt
import numpy as np
fig, axes = plt.subplots(nrows=2, ncols=2, sharey=True, sharex=True)
for i, ax in enumerate(axes.flat, start=1):
ax.set(aspect=1)
ax.plot(np.arange(0, i * 4, i))
plt.show()
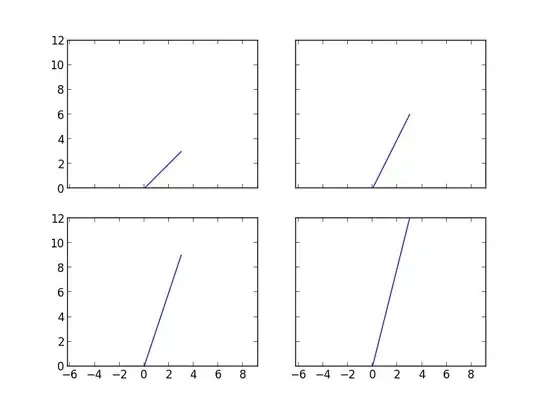
If you want them all to share their axes limits, but to have adjustable='box'
(i.e. non-square axes boundaries), use adjustable='box-forced'
:
import matplotlib.pyplot as plt
import numpy as np
fig, axes = plt.subplots(nrows=2, ncols=2, sharey=True, sharex=True)
for i, ax in enumerate(axes.flat, start=1):
ax.set(aspect=1, adjustable='box-forced', xticks=range(i))
ax.plot(np.arange(0, i * 4, i))
plt.show()
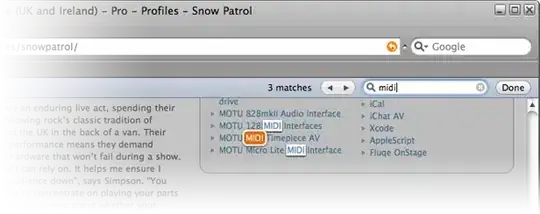
Edit: Sorry, I'm still a bit confused. Do you want something like this?
import matplotlib.pyplot as plt
import numpy as np
fig, axes = plt.subplots(nrows=2, ncols=2)
for i, ax in enumerate(axes.flat, start=1):
ax.set(adjustable='datalim', aspect=1)
ax.plot(np.arange(0, i * 4, i))
plt.show()
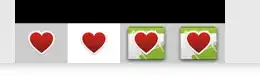
Okay, I think I finally understand your question. We both meant entirely different things by "aspect ratio".
In matplotlib, the aspect ratio of the plot refers to the relative scales of the data limits. In other words, if the aspect ratio of the plot is 1, a line with a slope of one will appear at 45 degrees. You were assuming that the aspect ratio applied to the outline of the axes and not the data plotted on the axes.
You just want the outline of the subplots to be square. (In which case, they all have different aspect ratios, as defined by matplotlib.)
In that case, you need a square figure. (There are other ways, but just making a square figure is far simpler. Matplotlib axes fill up a space that is proportional to the size of the figure they're in.)
import matplotlib.pyplot as plt
import numpy as np
# The key here is the figsize (it needs to be square). The position and size of
# axes in matplotlib are defined relative to the size of the figure.
fig, axes = plt.subplots(nrows=2, ncols=2, figsize=(8,8))
for i, ax in enumerate(axes.flat, start=1):
ax.plot(np.arange(0, i * 4, i))
# By default, subplots leave a bit of room for tick labels on the left.
# We'll remove it so that the axes are perfectly square.
fig.subplots_adjust(left=0.1)
plt.show()
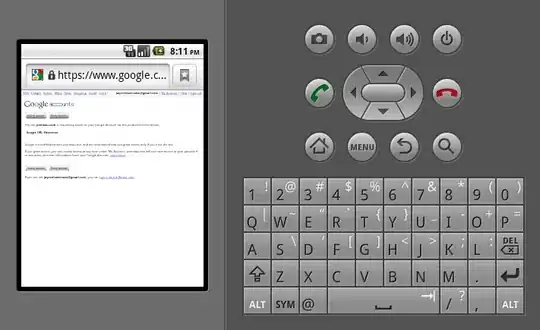