If one or more properties of an Artist need to be set to a specific value, matplotlib has a convenience method plt.setp
(can be used instead of a list comprehension).
plt.setp(ax.spines.values(), lw=0.2)
# or
plt.setp(ax.spines.values(), linewidth=0.2)
Another way is to simply use a loop. Each spine defines a set()
method that can be used to set a whole host of properties such as linewidth, alpha etc.
for side in ['top', 'bottom', 'left', 'right']:
ax.spines[side].set(lw=0.2)
A working example:
import matplotlib.pyplot as plt
x, y = [0, 1, 2], [0, 2, 1]
fig, ax = plt.subplots(figsize=(4, 2))
ax.plot(y)
ax.set(xticks=x, yticks=x, ylim=(0,2), xlim=(0,2));
plt.setp(ax.spines.values(), lw=5, color='red', alpha=0.2);
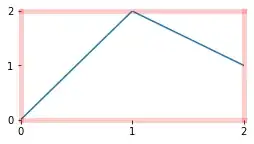