Do you mean save multiple figures into one file, or save multiple figures using one script?
Here's how you can save two different figures using one script.
>>> from matplotlib import pyplot as plt
>>> fig1 = plt.figure()
>>> plt.plot(range(10))
[<matplotlib.lines.Line2D object at 0x10261bd90>]
>>> fig2 = plt.figure()
>>> plt.plot(range(10,20))
[<matplotlib.lines.Line2D object at 0x10263b890>]
>>> fig1.savefig('fig1.png')
>>> fig2.savefig('fig2.png')
...which produces these two plots into their own ".png" files:
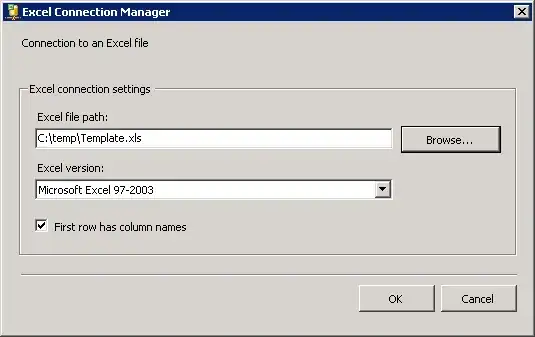
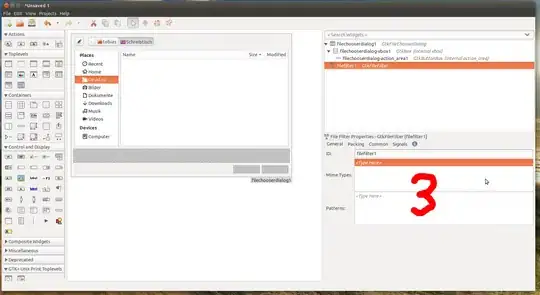
To save them to the same file, use subplots:
>>> from matplotlib import pyplot as plt
>>> fig = plt.figure()
>>> axis1 = fig.add_subplot(211)
>>> axis1.plot(range(10))
>>> axis2 = fig.add_subplot(212)
>>> axis2.plot(range(10,20))
>>> fig.savefig('multipleplots.png')
The above script produces this single ".png" file:
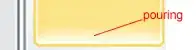