When creating an Intent you can pass explicit component name. i.e. class name. Only that component will now receive the intent.
example:
Intent myIntent = new Intent(getApplicationContext(),RequiredActivity.class);
startActivity(myIntent);
If you don't specify the exact component, Android will smartly let user choose one of the components that handles the intent.
example:
Intent myIntent = new Intent(Intent.ACTION_VIEW);
startActivity(myIntent);
If you want to go through all the components that handle the intent, yourself instead of letting android show choices to user, you can do that too:
example:
Intent myIntent = new Intent(Intent.ACTION_VIEW);
List<ResolveInfo> infoList = getPackageManager().queryIntentActivities(myIntent, 0);
for (ResolveInfo ri : infoList){
ActivityInfo ai = ri.activityInfo;
String packageName = ai.packageName;
String componentName = ai.name;
// you can pick up appropriate activity to start
// if(isAGoodMatch(packageName,componentName)){
// myIntent.setComponent(new ComponentName(packageName,componentName));
// startActivity(myIntent);
// break;
// }
}
I got six Activity matches for above code:
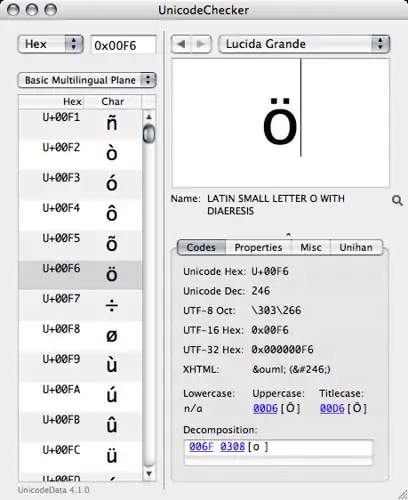